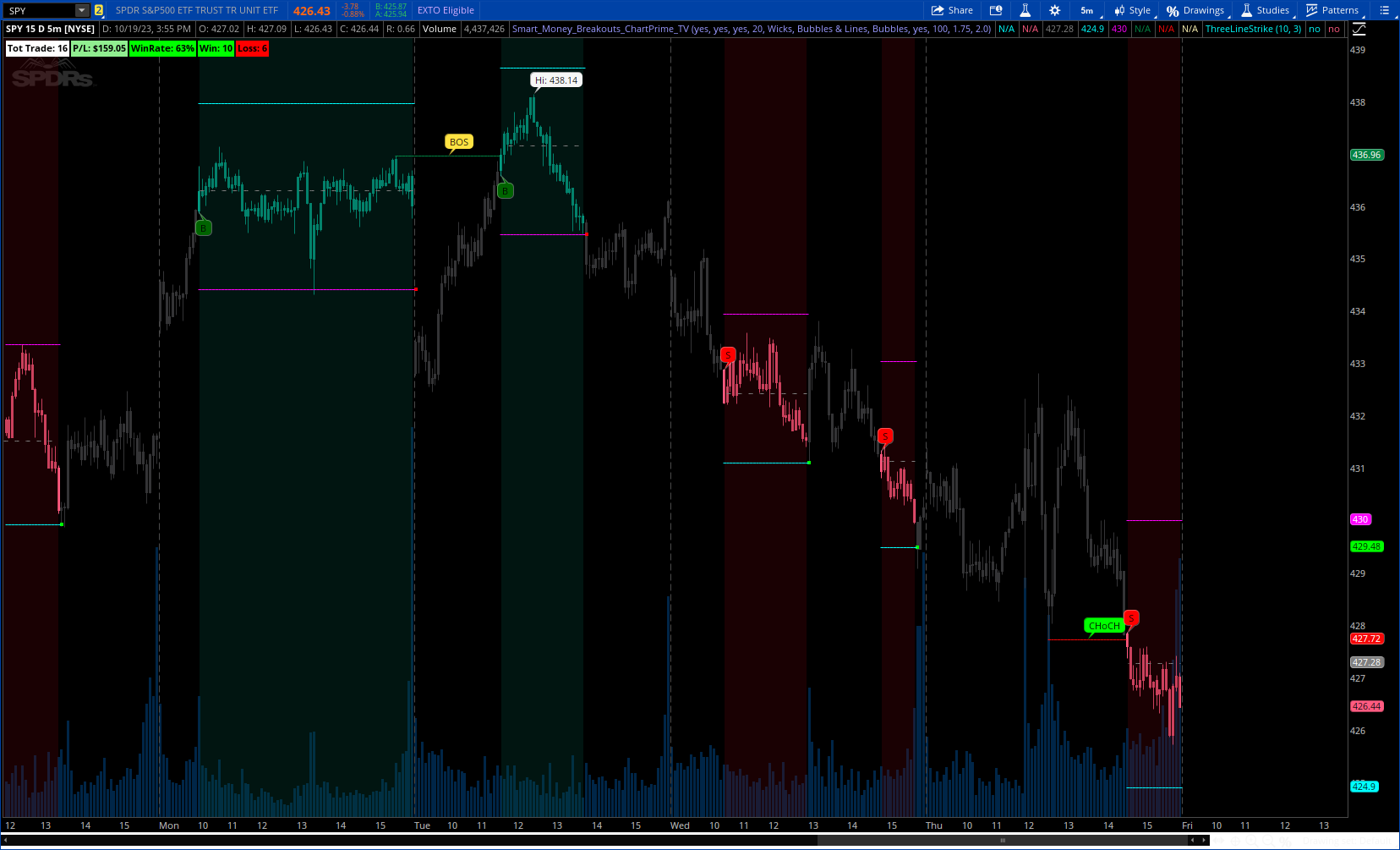
Author Message:
The "Smart Money Breakouts" indicator is designed to identify breakouts based on changes in character (CHOCH) or breaks of structure (BOS) patterns, facilitating automated trading with user-defined Take Profit (TP) level.
More Details : https://www.tradingview.com/v/aM3PRWEM/
CODE:
CSS:
# https://www.tradingview.com/v/aM3PRWEM/
#// This source code is subject to the terms of the Mozilla Public License 2.0 at
#// © ChartPrime
#indicator("Smart Money Breakouts [ChartPrime]", overlay=true, max_labels_count=500
# Converted and mod By Sam4Cok@Samer800 - 10 / 2023
input showInfoLabel = yes;
input colorBars = yes;
input colorBackground = yes;
input period = 20; #, 'Length',group = CORE,tooltip = "Swing Length")
input WicksOrBodyOfTheCandle = {default "Wicks", "Body"}; # "Wicks or Body of the candle"
input showBosChoch = {Default "Bubbles & Lines", "Bubbles Only", "Lines Only", "Don't Show"};
input signalType = {Default "Bubbles", "Arrows", "Don't Show"};
input showTargetLines = yes;
input LotSize = 100;
input ProfitMultiplyer = 1.75; # "Target Multiplyer "
input StopLossMultiplyer = 2.00;
def na = Double.NaN;
def pos = Double.POSITIVE_INFINITY;
def neg = Double.NEGATIVE_INFINITY;
def Sync = AbsValue(BarNumber());
def tar = ProfitMultiplyer * 10;
def stL = StopLossMultiplyer * 10;
def wick = WicksOrBodyOfTheCandle == WicksOrBodyOfTheCandle."Wicks";
def sigBubble = signalType == signalType."Bubbles";
def sigArrows = signalType == signalType."Arrows";
#method volAdj(int len)=>
def nATR = ATR(Length = 30);
def volAdj = Min(nATR * 0.3, close * (0.3 / 100));
def Adj = volAdj[20] / 2;
def bubble; def lines;
Switch (showBosChoch) {
Case "Bubbles Only" :
bubble = yes;
lines = no;
Case "Lines Only" :
bubble = no;
lines = yes;
Case "Don't Show" :
bubble = no;
lines = no;
Default :
bubble = yes;
lines = yes;
}
#-- Colors
DefineGlobalColor("GREEN", CreateColor(2, 133, 69));
DefineGlobalColor("TAIL", CreateColor(20, 141, 154));
DefineGlobalColor("RED" , CreateColor(246, 7, 7));
DefineGlobalColor("_Green", CreateColor(3, 156, 131));
DefineGlobalColor("_RED", GetColor(2));
DefineGlobalColor("dGreen", CreateColor(3,69,57));
DefineGlobalColor("dRed", CreateColor(86,3,10));
#-- Script
script VolCal {
input Index = 100;
input Sync = 50;
def Bars = AbsValue(Index - Sync);
def Green = fold i = 0 to Bars with p do
p + (if GetValue(close, i) > GetValue(open, i)
then GetValue(volume, i) else 0);
def Red = fold j = 0 to Bars with q do
q + (if GetValue(close, j) < GetValue(open, j)
then GetValue(volume, j) else 0);
def Total = fold v = 0 to Bars with l do
l + GetValue(volume, v);
def GreenRatio = Green / Total * 100;
def RedRatio = Red / Total * 100;
def VolCal = if GreenRatio > 55 then 1 else
if RedRatio > 55 then -1 else 0;
plot Out = VolCal;
}
script FindPivots {
input dat = close; # default data or study being evaluated
input HL = 0; # default high or low pivot designation, -1 low, +1 high
input lbL = 5; # default Pivot Lookback Left
input lbR = 1; # default Pivot Lookback Right
##############
def _nan; # used for non-number returns
def _BN; # the current barnumber
def _VStop; # confirms that the lookforward period continues the pivot trend
def _V; # the Value at the actual pivot point
def _pivotRange;
##############
_BN = BarNumber();
_nan = Double.NaN;
_pivotRange = lbL + lbL;
_VStop = if !IsNaN(dat[_pivotRange]) and lbR > 0 and lbL > 0 then
fold a = 1 to lbR + 1 with b=1 while b do
if HL > 0 then dat > GetValue(dat, -a) else dat < GetValue(dat, -a) else _nan;
if (HL > 0) {
_V = if _BN > lbL and dat == Highest(dat, lbL + 1) and _VStop
then dat else _nan;
} else {
_V = if _BN > lbL and dat == Lowest(dat, lbL + 1) and _VStop
then dat else _nan;
}
plot result = if !IsNaN(_V) and _VStop then _V else _nan;
}
def ph = findpivots(high, 1, period, period);
def pl = findpivots(low ,-1, period, period);
def nanPh = !isNaN(ph);
def nanPl = !isNaN(pl);
def fixPh = if nanPh then ph else fixPh[1];
def fixPl = if nanPl then pl else fixPl[1];
def ScrHigh = if wick then high else close;
def ScrLow = if wick then low else close;
def UpdatedHigh;
def UpdatedLow;
def ShortTrade;
def TradeisON;
def LongTrade;
def HighIndex;
def phActive;
def plActive;
def phActive_;
def plActive_;
def LowIndex;
def HBreak;
def TP;
def SL;
def BUY;
def SELL;
if nanPh {
UpdatedHigh = ph;
phActive_ = yes;
HighIndex = Sync;
} else {
UpdatedHigh = UpdatedHigh[1];
phActive_ = phActive[1];
HighIndex = HighIndex[1];
}
if nanPl {
UpdatedLow = pl;
plActive_ = yes;
LowIndex = Sync;
} else {
UpdatedLow = UpdatedLow[1];
plActive_ = plActive[1];
LowIndex = LowIndex[1];
}
#// LONG
if ScrHigh > UpdatedHigh and phActive_ {
BUY = yes;
phActive = no;
} else {
BUY = no;
phActive = phActive_;
}
#//Sell
if ScrLow < UpdatedLow and plActive_ {
SELL = yes;
plActive = no;
} else {
SELL = no;
plActive = plActive_;
}
#// lets Draw
def barB;def LabLocB;def labB;
def barS;def LabLocS;def labS;
if BUY and !TradeisON[1] {
barB = Sync;
barS = barS[1];
LabLocB = floor(Sync - (Sync - HighIndex) / 2);
LabLocS = LabLocS[1];
labB = if !HBreak[1] then 1 else -1;
labS = labS[1];
HBreak = yes;
} else
if SELL and !TradeisON[1] {
barB = barB[1];
barS = Sync;
LabLocB = LabLocB[1];
LabLocS = floor(Sync - (Sync - LowIndex) / 2);
labB = labB[1];
labS = if HBreak[1] then 1 else -1;
HBreak = no;
} else {
barB = barB[1];
barS = barS[1];
LabLocB = LabLocB[1];
LabLocS = LabLocS[1];
labB = labB[1];
labS = labS[1];
HBreak = HBreak[1];
}
def Long = BUY and !TradeisON[1];
def Short = SELL and !TradeisON[1];
def TradeFire = Long or Short;
if Long and !TradeisON[1] {
LongTrade = yes;
ShortTrade = no;
} else
if Short and !TradeisON[1] {
LongTrade = no;
ShortTrade = yes;
} else {
LongTrade = LongTrade[1];
ShortTrade = ShortTrade[1];
}
def win; def los;def entry;
def profit; def losses;
if TradeFire and !TradeisON[1] {
entry = ohlc4[-1];
TP = if Long then entry + (Adj * Tar) else
if Short then entry - (Adj * Tar) else na;
SL = if Long then entry - (Adj * stL) else
if Short then entry + (Adj * stL) else na;
win = if !sync then 0 else win[1];
los = if !sync then 0 else los[1];
profit = profit[1];
losses = losses[1];
TradeisON = yes;
} else
if LongTrade and TradeisON[1] {
entry = entry[1];
TP = TP[1];
SL = SL[1];
win = if high >= TP then win[1] + 1 else win[1];
los = if close <= SL then los[1] + 1 else los[1];
profit = if high crosses above TP then profit[1] + AbsValue(close - Entry) * lotSize else profit[1];
losses = if close crosses below SL then losses[1] + AbsValue(Entry - close) * lotSize else losses[1];
TradeisON = if high >= TP then no else
if close <= SL then no else TradeisON[1];
} else
if ShortTrade and TradeisON[1] {
entry = entry[1];
TP = TP[1];
SL = SL[1];
win = if low <= TP then win[1] + 1 else win[1];
los = if close >= SL then los[1] + 1 else los[1];
profit = if low crosses below TP then profit[1] + AbsValue(entry - close) * lotSize else profit[1];
losses = if close Crosses Above SL then losses[1] + AbsValue(close - Entry) * lotSize else losses[1];
TradeisON = if low <= tp then no else
if close >= SL then no else TradeisON[1];
} else {
entry = na;
TP = TP[1];
SL = SL[1];
win = if !sync then 0 else win[1];
los = if !sync then 0 else los[1];
profit = profit[1];
losses = losses[1];
TradeisON = TradeisON[1];
}
def volH = VolCal(HighIndex,Sync);
def volL = VolCal(LowIndex,Sync);
def BearCon = TradeisON and ShortTrade;
def BullCon = TradeisON and LongTrade;
def signH = if BUY and !TradeisON then volH else signH[1];
def signS = if SELL and !TradeisON then volL else signS[1];
def buyCond = BullCon and !BullCon[1];
def sellCond = BearCon and !BearCon[1];
def barBuy = if buyCond then HighIndex -1 else barBuy[1];
def barSell = if sellCond then lowIndex -1 else barSell[1];
#-- Signals
plot arrUp = if sigArrows and buyCond then low else na;
plot arrDn = if sigArrows and sellCond then high else na;
arrUp.SetPaintingStrategy(PaintingStrategy.BOOLEAN_ARROW_UP);
arrDn.SetPaintingStrategy(PaintingStrategy.BOOLEAN_ARROW_DOWN);
arrUp.AssignValueColor(if signH>0 then color.CYAN else Color.VIOLET);
arrDn.AssignValueColor(if signS<0 then color.MAGENTA else Color.PLUM);
AddchartBubble(sigBubble and buyCond, low,"B" ,if signH>0 then color.GREEN else Color.DARK_GREEN, no);
AddchartBubble(sigBubble and sellCond, high,"S",if signS<0 then color.RED else Color.DARK_RED);
#-- Trade
plot entryLine = if !showTargetLines then na else
if TradeisON then entry else na;
entryLine.SetDefaultColor(Color.GRAY);
entryLine.SetStyle(Curve.SHORT_DASH);
plot lineUp = if !showTargetLines then na else
if BullCon then tp else
if BearCon then tp else na;
plot lineDn = if !showTargetLines then na else
if BullCon then sl else
if BearCon then sl else na;
lineUp.SetDefaultColor(Color.CYAN);
lineDn.SetDefaultColor(Color.MAGENTA);
lineUp.SetPaintingStrategy(PaintingStrategy.HORIZONTAL);
lineDn.SetPaintingStrategy(PaintingStrategy.HORIZONTAL);
def plotUp = lines and highestAll(barB) > Sync and Sync > highestAll(barBuy);
def plotDn = lines and highestAll(barS) > Sync and Sync > highestAll(barSell);
plot BullPvt = if plotUp then if fixPh then fixPh else na else na;
plot BearPvt = if plotDn then if fixPl then fixPl else na else na;
BullPvt.SetStyle(Curve.LONG_DASH);
BearPvt.SetStyle(Curve.LONG_DASH);
BullPvt.SetDefaultColor(GlobalColor("Green"));
BearPvt.SetDefaultColor(GlobalColor("Red"));
BullPvt.SetPaintingStrategy(PaintingStrategy.HORIZONTAL);
BearPvt.SetPaintingStrategy(PaintingStrategy.HORIZONTAL);
def bubUpCond = bubble and highestAll(LabLocB) == sync;
def bubDnpCond = bubble and highestAll(LabLocS) == sync;
AddChartBubble(bubUpCond, fixPh ,if labB > 0 then "BOS" else "CHoCH",
if signH>0 then Color.GREEN else if signH < 0 then Color.RED else GetColor(4));
AddChartBubble(bubDnpCond, fixPl ,if labS > 0 then "BOS" else "CHoCH",
if signS<0 then Color.GREEN else if signS > 0 then Color.RED else GetColor(4));
#-- BG and Bar Color
AddCloud(if !colorBackground then na else if BullCon then pos else na, neg, GlobalColor("dGreen"));
AddCloud(if !colorBackground then na else if BearCon then pos else na, neg, GlobalColor("dRed"));
AssignPriceColor(if !colorBars then Color.CURRENT else
if BearCon then GlobalColor("_RED") else
if BullCon then GlobalColor("_Green") else CreateColor(52, 52, 54));
#--
plot TradeEnd = if !TradeisON and TradeisON[1] then
if los>los[1] then lineDn[1] else lineUp[1] else na;
TradeEnd.AssignValueColor(if win>win[1] then Color.GREEN else Color.RED);
TradeEnd.SetPaintingStrategy(PaintingStrategy.SQUARES);
#-- Label
def totTrade = win + los;
def winRate = Round(win / totTrade * 100, 0);
def PandL = Round(profit - losses, 2);
AddLabel(showInfoLabel, "Tot Trade: " + totTrade, color.WHITE);
AddLabel(showInfoLabel, "P/L: $" + PandL,
if PandL > 0 then Color.LIGHT_GREEN else
if PandL < 0 then Color.PINK else Color.GRAY);
AddLabel(showInfoLabel, "WinRate: " + winRate + "%",
if winRate > 50 then Color. Green else
if winRate < 50 then color.RED else Color.GRAY);
AddLabel(showInfoLabel, "Win: " + win, color.GREEN);
AddLabel(showInfoLabel, "Loss: " + los, color.RED);
#-- END of CODE