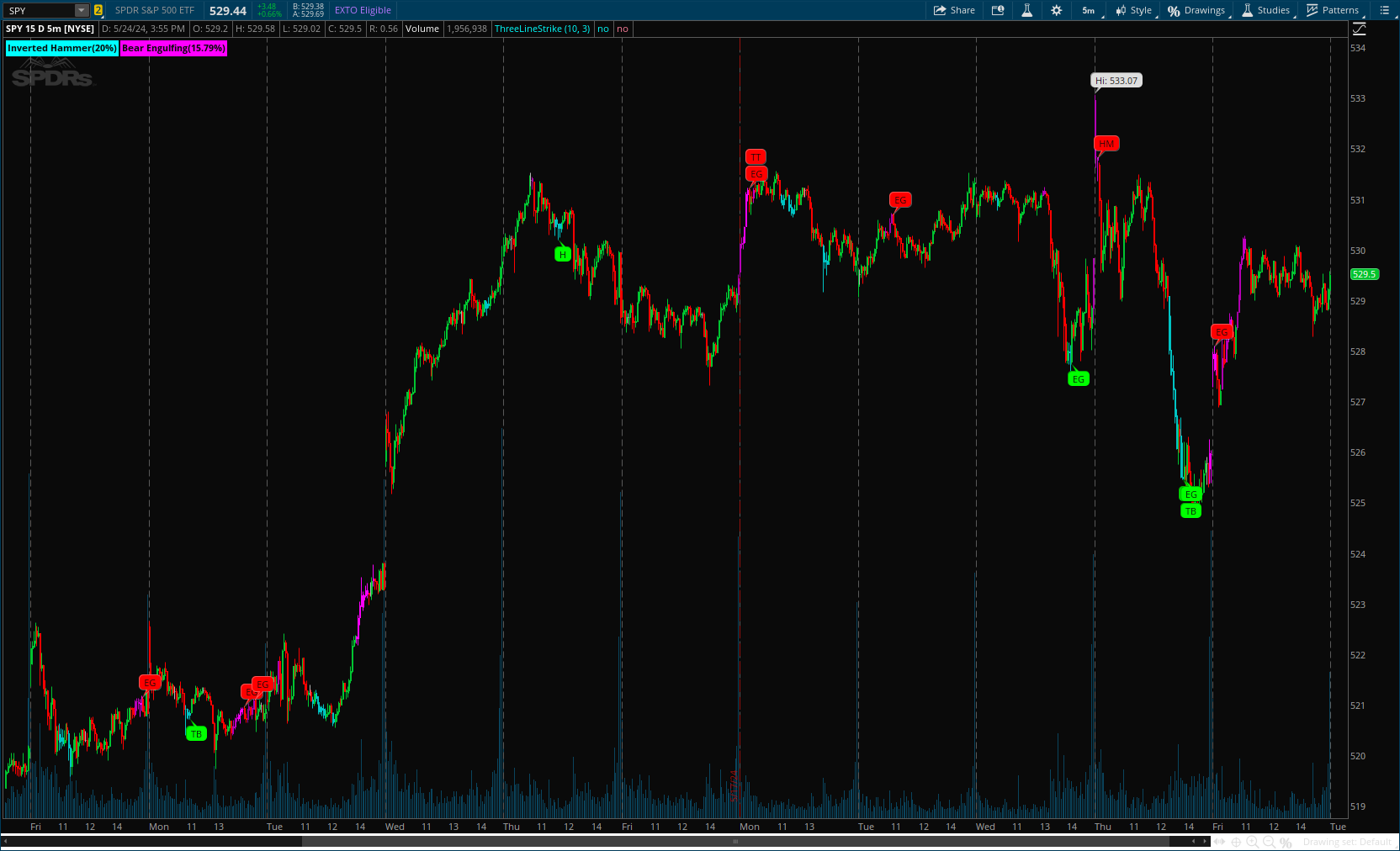
Author Message: (I added option for MTF and Stoch or Stoch RSI).
The Reversal Candlestick Structure indicator detects multiple candlestick patterns occurring when trends are most likely to experience a reversal in real-time. The reversal detection method includes various settings allowing users to adjust the reversal detection algorithm more precisely.
A dashboard showing the percentage of patterns detected as reversals is also included.
CODE:
CSS:
#/ This work is licensed under a Attribution-NonCommercial-ShareAlike 4.0 International (CC BY-NC-SA 4.0) h
#// © LuxAlgo
#indicator("Reversal Candlestick Structure [LuxAlgo]",shorttitle = "LuxAlgo - Reversal Candlestick Structure"
# Converted by Sam4Cok@Samer800 - 05/2024
#//Bullish
input timeframe = {Default "Chart", "Custom Timeframe"};
input customTimeframe = AggregationPeriod.FIFTEEN_MIN;
input labelOptions = {Default "Show Top Reversal Only", "Show All Reversal Structure", "Don't Show Labels"};
input calcMethod = {"Stochastic", Default "Stochastic RSI"};
input TrendLength = 14; #, 'Trend Length'
input threshold = 80; #, 'Threshold', minval = 0, maxval = 100
input WarmupLength = 20; #, 'Warmup Length',
input CandlesColorStyle = {Default "Only Threshold Candles", "Color All Candles", "Don't Color Candles"};
input BullishHammer = yes;
input BullishInvertedHammer = yes;
input BullishEngulfing = yes;
input BullishRisingThree = yes;
input BullishThreeWhiteSoldiers = yes;
input BullishMorningStar = yes;
input BullishHarami = yes;
input BullishTweezerBottom = yes;
#//Bearish
input BearishHangingMan = yes;
input BearishShootingStar = yes;
input BearishEngulfing = yes;
input BearishFallingThree = yes;
input BearishThreeBlackCrows = yes;
input BearishEveningStar = yes;
input BearishHarami = yes;
input BearishTweezerTop = yes;
def na = Double.NaN;
def dash = labelOptions==labelOptions."Show All Reversal Structure";
def top = labelOptions==labelOptions."Show Top Reversal Only";
def all = CandlesColorStyle==CandlesColorStyle."Color All Candles";
def bcTog = CandlesColorStyle==CandlesColorStyle."Don't Color Candles";
def sto = calcMethod==calcMethod."Stochastic";
def hammerTog = BullishHammer;
def ihammerTog = BullishInvertedHammer;
def bulleTog = BullishEngulfing;
def r3Tog = BullishRisingThree;
def twsTog = BullishThreeWhiteSoldiers;
def mstarTog = BullishMorningStar;
def bullhTog = BullishHarami;
def btmTweezTog = BullishTweezerBottom;
def hmanTog = BearishHangingMan;
def sstarTog = BearishShootingStar;
def beareTog = BearishEngulfing;
def f3Tog = BearishFallingThree;
def tbcTog = BearishThreeBlackCrows;
def estarTog = BearishEveningStar;
def bearhTog = BearishHarami;
def topTweezTog = BearishTweezerTop;
def h;
def l;
def c;
def o;
def hl;
Switch (timeframe) {
Case "Custom Timeframe" :
h = high(Period = customTimeframe);
l = low(Period = customTimeframe);
c = close(Period = customTimeframe);
o = open(Period = customTimeframe);
hl = hl2(Period = customTimeframe);
Default :
h = high;
l = low;
c = close;
o = open;
hl = hl2;
}
#//Functions
# stoch(source, high, low, length) =>
script stoch {
input src = close;
input h = high;
input l = low;
input len = 14;
def hh = Highest(h, len);
def ll = Lowest(l, len);
def c1 = src - ll;
def c2 = hh - ll;
def stoch = if c2 != 0 then c1 / c2 * 100 else 0;
plot return = stoch;
}
script count {
input condition = yes;
input filter = yes;
def reversals = reversals[1] + (if condition and filter then 1 else 0);
def total = total[1] + (if condition then 1 else 0);
def percentChg = reversals / total * 100;
def bothCond = condition and filter;
def rndPer = Round(percentChg, 2);
plot cond = if isNaN(bothCond) then no else bothCond;
plot per = if isNaN(rndPer) then 0 else rndPer;
plot cnt = if isNaN(total) then 0 else total;
}
#//Trend Detection
def rsi = rsi(Price = c, Length = TrendLength);
def calSrc = if sto then c else rsi;
def k = stoch(calSrc, calSrc, calSrc, TrendLength);
def alpha = 2 / (WarmupLength + 1);
def smooth_k = if isNaN(smooth_k[1]) then 0 else
if k > 50 then smooth_k[1] + (100 - smooth_k[1]) * alpha else
if k < 50 then smooth_k[1] + (0 - smooth_k[1]) * alpha else k;
def is_bullish = k >= threshold and smooth_k >= threshold;
def is_bearish = k <= 100 - threshold and smooth_k <= 100 - threshold;
#//Candestick Patterns
def rc = c < o; # // Red Candle
def gc = c > o; # // Green Candle
#//Candle measurements
def c_top = Max(o, c); # //Top of candle
def c_bot = Min(o, c); # //Bottom of candle
def hl_width = h - l; # //Total candle width (wick to wick)
def bod_width = (c_top - c_bot); # //Width of candle body (open to close)
def hw_per = ((h - c_top) / hl_width) * 100; # //Percent of total candle width that is occupied by the upper wick
def lw_per = ((c_bot - l) / hl_width) * 100; # //Percent of total candle width that is occupied by the lower wick
def b_per = (bod_width / hl_width) * 100; # //Percent of total candle width that is occupied by the candle body
def doji = Round(c, 2) == Round(o, 2);
#//Bullish patterns
def hammer_ = count((lw_per > (b_per * 2) and b_per < 50 and hw_per < 2 and !doji), is_bearish).cond;
def hammer_per = count((lw_per > (b_per * 2) and b_per < 50 and hw_per < 2 and !doji), is_bearish).per;
def hammer_count = count((lw_per > (b_per * 2) and b_per < 50 and hw_per < 2 and !doji), is_bearish).cnt;
def inv_hammer = count((hw_per > (b_per * 2) and b_per < 50 and lw_per < 2 and !doji), is_bearish).cond;
def inv_hammer_per = count((hw_per > (b_per * 2) and b_per < 50 and lw_per < 2 and !doji), is_bearish).per;
def inv_hammer_count = count((hw_per > (b_per * 2) and b_per < 50 and lw_per < 2 and !doji), is_bearish).cnt;
def rising_3 = count((gc[4] and b_per[4] > 50)
and (rc[3] and c_top[3] <= h[4] and c_bot[3] >= l[4])
and (rc[2] and c_top[2] <= h[4] and c_bot[2] >= l[4])
and (rc[1] and c_top[1] <= h[4] and c_bot[1] >= l[4])
and (gc and c > h[4] and b_per > 50), is_bearish[1]).cond;
def rising_3_per = count((gc[4] and b_per[4] > 50)
and (rc[3] and c_top[3] <= h[4] and c_bot[3] >= l[4])
and (rc[2] and c_top[2] <= h[4] and c_bot[2] >= l[4])
and (rc[1] and c_top[1] <= h[4] and c_bot[1] >= l[4])
and (gc and c > h[4] and b_per > 50), is_bearish[1]).per;
def rising_3_count = count((gc[4] and b_per[4] > 50)
and (rc[3] and c_top[3] <= h[4] and c_bot[3] >= l[4])
and (rc[2] and c_top[2] <= h[4] and c_bot[2] >= l[4])
and (rc[1] and c_top[1] <= h[4] and c_bot[1] >= l[4])
and (gc and c > h[4] and b_per > 50), is_bearish[1]).cnt;
def bull_engulfing = count(rc[1] and gc and (bod_width > (bod_width[1] / 2)) and
(o < c[1]) and c_top > c_top[1] and (!rising_3) and (!doji[1]), is_bearish[1]).cond;
def bull_engulfing_per = count(rc[1] and gc and (bod_width > (bod_width[1] / 2)) and
(o < c[1]) and c_top > c_top[1] and (!rising_3) and (!doji[1]), is_bearish[1]).per;
def bull_engulfing_count = count(rc[1] and gc and (bod_width > (bod_width[1] / 2)) and
(o < c[1]) and c_top > c_top[1] and (!rising_3) and (!doji[1]), is_bearish[1]).cnt;
def soldiers = count((gc[2] and b_per[2] > 70) and
(gc[1] and b_per[1] > 70 and c_bot[1] >= c_bot[2] and c_bot[1] <= c_top[2] and c[1] > h[2]) and
(gc and b_per > 70 and c_bot >= c_bot[1] and c_bot <= c_top[1] and c > h[1]), is_bearish[3]).cond;
def soldiers_per = count((gc[2] and b_per[2] > 70) and
(gc[1] and b_per[1] > 70 and c_bot[1] >= c_bot[2] and c_bot[1] <= c_top[2] and c[1] > h[2]) and
(gc and b_per > 70 and c_bot >= c_bot[1] and c_bot <= c_top[1] and c > h[1]), is_bearish[3]).per;
def soldiers_count = count((gc[2] and b_per[2] > 70) and
(gc[1] and b_per[1] > 70 and c_bot[1] >= c_bot[2] and c_bot[1] <= c_top[2] and c[1] > h[2]) and
(gc and b_per > 70 and c_bot >= c_bot[1] and c_bot <= c_top[1] and c > h[1]), is_bearish[3]).cnt;
def m_star = count((rc[2] and b_per[2] > 80) and
(rc[1] and bod_width[1] < (bod_width[2] / 2) and o[1] < c[2]) and
(gc and c > hl[2]), is_bearish).cond;
def m_star_per = count((rc[2] and b_per[2] > 80) and
(rc[1] and bod_width[1] < (bod_width[2] / 2) and o[1] < c[2]) and
(gc and c > hl[2]), is_bearish).per;
def m_star_count = count((rc[2] and b_per[2] > 80) and
(rc[1] and bod_width[1] < (bod_width[2] / 2) and o[1] < c[2]) and
(gc and c > hl[2]), is_bearish).cnt;
def bull_harami = count(gc and (h <= c_top[1] and l >= c_bot[1]) and rc[1], is_bearish).cond;
def bull_harami_per = count(gc and (h <= c_top[1] and l >= c_bot[1]) and rc[1], is_bearish).per;
def bull_harami_count = count(gc and (h <= c_top[1] and l >= c_bot[1]) and rc[1], is_bearish).cnt;
def tweezer_btm = count((Round(l, 2) - Round(l[1], 2)) == 0 and gc and rc[1], is_bearish[1]).cond;
def tweezer_btm_per = count((Round(l, 2) - Round(l[1], 2)) == 0 and gc and rc[1], is_bearish[1]).per;
def tweezer_btm_count = count((Round(l, 2) - Round(l[1], 2)) == 0 and gc and rc[1], is_bearish[1]).cnt;
#// Bearish patterns
def s_star = count((hw_per > (b_per * 2) and b_per < 50 and lw_per < 2 and !doji), is_bullish).cond;
def s_star_per = count((hw_per > (b_per * 2) and b_per < 50 and lw_per < 2 and !doji), is_bullish).per;
def s_star_count = count((hw_per > (b_per * 2) and b_per < 50 and lw_per < 2 and !doji), is_bullish).cnt;
def h_man = count((lw_per > (b_per * 2) and b_per < 50 and hw_per < 2 and !doji), is_bullish).cond;
def h_man_per = count((lw_per > (b_per * 2) and b_per < 50 and hw_per < 2 and !doji), is_bullish).per;
def h_man_count = count((lw_per > (b_per * 2) and b_per < 50 and hw_per < 2 and !doji), is_bullish).cnt;
def falling_3 = count((rc[4] and b_per[4] > 50)
and (gc[3] and c_top[3] <= h[4] and c_bot[3] >= l[4])
and (gc[2] and c_top[2] <= h[4] and c_bot[2] >= l[4])
and (gc[1] and c_top[1] <= h[4] and c_bot[1] >= l[4])
and (rc and c < l[4] and b_per > 50), is_bullish[1]).cond;
def falling_3_per = count((rc[4] and b_per[4] > 50)
and (gc[3] and c_top[3] <= h[4] and c_bot[3] >= l[4])
and (gc[2] and c_top[2] <= h[4] and c_bot[2] >= l[4])
and (gc[1] and c_top[1] <= h[4] and c_bot[1] >= l[4])
and (rc and c < l[4] and b_per > 50), is_bullish[1]).per;
def falling_3_count = count((rc[4] and b_per[4] > 50)
and (gc[3] and c_top[3] <= h[4] and c_bot[3] >= l[4])
and (gc[2] and c_top[2] <= h[4] and c_bot[2] >= l[4])
and (gc[1] and c_top[1] <= h[4] and c_bot[1] >= l[4])
and (rc and c < l[4] and b_per > 50), is_bullish[1]).cnt;
def bear_engulfing = count(gc[1] and rc and (bod_width > (bod_width[1]/2)) and
(o > c[1]) and c_bot < c_bot[1] and (!falling_3) and (!doji[1]), is_bullish[1]).cond;
def bear_engulfing_per = count(gc[1] and rc and (bod_width > (bod_width[1]/2)) and
(o > c[1]) and c_bot < c_bot[1] and (!falling_3) and (!doji[1]), is_bullish[1]).per;
def bear_engulfing_count = count(gc[1] and rc and (bod_width > (bod_width[1]/2)) and
(o > c[1]) and c_bot < c_bot[1] and (!falling_3) and (!doji[1]), is_bullish[1]).cnt;
def crows = count((rc[2] and b_per[2]>70) and (rc[1] and b_per[1]>70 and
c_top[1] <= c_top[2] and c_top[1] >= c_bot[2] and c[1] < l[2]) and
(rc and b_per>70 and c_top <= c_top[1] and c_top >= c_bot[1] and c < l[1]), is_bullish[3]).cond;
def crows_per = count((rc[2] and b_per[2]>70) and (rc[1] and b_per[1]>70 and
c_top[1] <= c_top[2] and c_top[1] >= c_bot[2] and c[1] < l[2]) and
(rc and b_per>70 and c_top <= c_top[1] and c_top >= c_bot[1] and c < l[1]), is_bullish[3]).per;
def crows_count = count((rc[2] and b_per[2]>70) and (rc[1] and b_per[1]>70 and
c_top[1] <= c_top[2] and c_top[1] >= c_bot[2] and c[1] < l[2]) and
(rc and b_per>70 and c_top <= c_top[1] and c_top >= c_bot[1] and c < l[1]), is_bullish[3]).cnt;
def e_star = count((gc[2] and b_per[2] > 80) and
(gc[1] and bod_width[1] < (bod_width[2]/2) and o[1] > c[2]) and
(rc and c < hl[2]), is_bullish).cond;
def e_star_per = count((gc[2] and b_per[2] > 80) and
(gc[1] and bod_width[1] < (bod_width[2]/2) and o[1] > c[2]) and
(rc and c < hl[2]), is_bullish).per;
def e_star_count = count((gc[2] and b_per[2] > 80) and
(gc[1] and bod_width[1] < (bod_width[2]/2) and o[1] > c[2]) and
(rc and c < hl[2]), is_bullish).cnt;
def bear_harami = count(rc and (h <= c_top[1] and l >= c_bot[1]) and gc[1], is_bullish).cond;
def bear_harami_per = count(rc and (h <= c_top[1] and l >= c_bot[1]) and gc[1], is_bullish).per;
def bear_harami_count = count(rc and (h <= c_top[1] and l >= c_bot[1]) and gc[1], is_bullish).cnt;
def tweezer_top = count(Round(h, 2) - Round(h[1], 2) == 0 and rc and gc[1], is_bullish[1]).cond;
def tweezer_top_per = count(Round(h, 2) - Round(h[1], 2) == 0 and rc and gc[1], is_bullish[1]).cond;
def tweezer_top_count = count(Round(h, 2) - Round(h[1], 2) == 0 and rc and gc[1], is_bullish[1]).cond;
#//Labels
#//Bullish patterns
AddChartBubble(hammerTog and hammer_ and !hammer_[1], low, "H", color.GREEN, no);
AddChartBubble(ihammerTog and inv_hammer and !inv_hammer[1], low, "IH", color.GREEN, no);
AddChartBubble(sstarTog and s_star and !s_star[1], high, "SS", color.RED);
AddChartBubble(hmanTog and h_man and !h_man[1], high, "HM", color.RED);
AddChartBubble(r3Tog and rising_3 and !rising_3[1], low, "R3", color.GREEN, no);
AddChartBubble(f3Tog and falling_3 and !falling_3[1], high, "F3", color.RED);
AddChartBubble(bulleTog and bull_engulfing and !bull_engulfing[1], low, "EG", color.GREEN, no);
AddChartBubble(btmTweezTog and tweezer_btm and !tweezer_btm[1], low, "TB", color.GREEN, no);
#//Bearish patterns
AddChartBubble(beareTog and bear_engulfing and !bear_engulfing[1], high, "EG", color.RED);
AddChartBubble(twsTog and soldiers and !soldiers[1], low, "3WS", color.GREEN, no);
AddChartBubble(tbcTog and crows and !crows[1], high, "3BC", color.RED);
AddChartBubble(mstarTog and m_star and !m_star[1], low, "MS", color.GREEN, no);
AddChartBubble(estarTog and e_star and !e_star[1], high, "ES", color.RED);
AddChartBubble(bullhTog and bull_harami and !bull_harami[1], low, "H", color.GREEN, no);
AddChartBubble(bearhTog and bear_harami and !bear_harami[1], high, "H", color.RED);
AddChartBubble(topTweezTog and tweezer_top and !tweezer_top[1], high, "TT", color.RED);
#def col = if
def smthk = if k > threshold then smthk[1] + (100 - smthk[1]) * alpha else
if k < (100 - threshold) then smthk[1] + (0 - smthk[1]) * alpha else k;
def col = if isNaN(smthk) then 54 else
if smthk > threshold then 250 else
if smthk < (100 - smthk) then 54 else smthk * 2.5;
# if isNaN(smooth_k) then 0 else
# if smooth_k > 100 then 100 else if smooth_k < 0 then 0 else smooth_k;
def css = if is_bullish then -1 else if is_bearish then 1 else 0;
def colDn = if col <= threshold then 32 else
if col >= 100 then 250 else col * 2.5;
def colUp = if col <= 0 then 250 else
if col >= (100 - threshold) then 32 else 250 - (col * 2.5);
AssignPriceColor(if bcTog then Color.CURRENT else
# if all then CreateColor(col * 2.5, 250 - col * 2.5, 250 - col * 2.5) else
if all then CreateColor(col ,250 - col, 0) else
if css > 0 then CreateColor(0,250 - col ,250 - col ) else
if css < 0 then CreateColor(col,0 , col) else Color.CURRENT);
#// Bullish Stats
AddLabel(dash and hammer_per, "Hammer(" + hammer_per + "%)", Color.GREEN);
AddLabel(dash and inv_hammer_per, "Inverted Hammer(" + inv_hammer_per + "%)", Color.GREEN);
AddLabel(dash and bull_engulfing_per, "Bullish Engulfing(" + bull_engulfing_per + "%)", Color.GREEN);
AddLabel(dash and rising_3_per, "Rising 3(" + rising_3_per + "%)", Color.GREEN);
AddLabel(dash and soldiers_per, "3 White Soldiers(" + soldiers_per + "%)", Color.GREEN);
AddLabel(dash and m_star_per, "Morning Star(" + m_star_per + "%)", Color.GREEN);
AddLabel(dash and bull_harami_per, "Bull Harami(" + bull_harami_per + "%)", Color.GREEN);
AddLabel(dash and tweezer_btm_per, "Tweezer Bottom(" + tweezer_btm_per + "%)", Color.GREEN);
# // Bearish Stats
AddLabel(dash and h_man_per, "Hanging Man(" + h_man_per + "%)", Color.RED);
AddLabel(dash and s_star_per, "Shooting Star(" + s_star_per + "%)", Color.RED);
AddLabel(dash and bear_engulfing_per, "Bear Engulfing(" + bear_engulfing_per + "%)", Color.RED);
AddLabel(dash and falling_3_per, "Falling 3(" + falling_3_per + "%)", Color.RED);
AddLabel(dash and crows_per, "3 Black Crows(" + crows_per + "%)", Color.RED);
AddLabel(dash and e_star_per, "Evening Star(" + e_star_per + "%)", Color.RED);
AddLabel(dash and bear_harami_per, "Bear Harami(" + bear_harami_per + "%)", Color.RED);
AddLabel(dash and tweezer_top_per, "Tweezer Top(" + tweezer_top_per + "%)", Color.RED);
script Ranking {
input v0 = 0;
input v1 = 0;
input v2 = 0;
input v3 = 0;
input v4 = 0;
input v5 = 0;
input v6 = 0;
input v7 = 0;
input v8 = 0;
def r1 = v0 > v1;
def r2 = v0 > v2;
def r3 = v0 > v3;
def r4 = v0 > v4;
def r5 = v0 > v5;
def r6 = v0 > v6;
def r7 = v0 > v7;
def r8 = v0 > v8;
plot result = r1 + r2 + r3 + r4 + r5 + r6 + r7 + r8 + 1;
}
#-- Bull
def ham = Ranking(hammer_per, hammer_per, inv_hammer_per, bull_engulfing_per, rising_3_per, soldiers_per, m_star_per, bull_harami_per, tweezer_btm_per);
def inv = Ranking(inv_hammer_per, hammer_per, inv_hammer_per, bull_engulfing_per, rising_3_per, soldiers_per, m_star_per, bull_harami_per, tweezer_btm_per);
def bEng = Ranking(bull_engulfing_per, hammer_per, inv_hammer_per, bull_engulfing_per, rising_3_per, soldiers_per, m_star_per, bull_harami_per, tweezer_btm_per);
def rsi3 = Ranking(rising_3_per, hammer_per, inv_hammer_per, bull_engulfing_per, rising_3_per, soldiers_per, m_star_per, bull_harami_per, tweezer_btm_per);
def sold = Ranking(soldiers_per, hammer_per, inv_hammer_per, bull_engulfing_per, rising_3_per, soldiers_per, m_star_per, bull_harami_per, tweezer_btm_per);
def mstar = Ranking(m_star_per, hammer_per, inv_hammer_per, bull_engulfing_per, rising_3_per, soldiers_per, m_star_per, bull_harami_per, tweezer_btm_per);
def bHar = Ranking(bull_harami_per, hammer_per, inv_hammer_per, bull_engulfing_per, rising_3_per, soldiers_per, m_star_per, bull_harami_per, tweezer_btm_per);
def TwzB = Ranking(tweezer_btm_per, hammer_per, inv_hammer_per, bull_engulfing_per, rising_3_per, soldiers_per, m_star_per, bull_harami_per, tweezer_btm_per);
#-- bear
def hang = Ranking(h_man_per, h_man_per, s_star_per, bear_engulfing_per, falling_3_per, crows_per, e_star_per, bear_harami_per, tweezer_top_per);
def sstar = Ranking(s_star_per, h_man_per, s_star_per, bear_engulfing_per, falling_3_per, crows_per, e_star_per, bear_harami_per, tweezer_top_per);
def sEng = Ranking(bear_engulfing_per, h_man_per, s_star_per, bear_engulfing_per, falling_3_per, crows_per, e_star_per, bear_harami_per, tweezer_top_per);
def fall3 = Ranking(falling_3_per, h_man_per, s_star_per, bear_engulfing_per, falling_3_per, crows_per, e_star_per, bear_harami_per, tweezer_top_per);
def crow = Ranking(crows_per, h_man_per, s_star_per, bear_engulfing_per, falling_3_per, crows_per, e_star_per, bear_harami_per, tweezer_top_per);
def estar = Ranking(e_star_per, h_man_per, s_star_per, bear_engulfing_per, falling_3_per, crows_per, e_star_per, bear_harami_per, tweezer_top_per);
def sHar = Ranking(bear_harami_per, h_man_per, s_star_per, bear_engulfing_per, falling_3_per, crows_per, e_star_per, bear_harami_per, tweezer_top_per);
def TwzT = Ranking(tweezer_top_per, h_man_per, s_star_per, bear_engulfing_per, falling_3_per, crows_per, e_star_per, bear_harami_per, tweezer_top_per);
def maxBull = Max(ham, Max(inv, Max(bEng, Max(rsi3, Max(sold, Max(mstar, Max(bHar, TwzB)))))));
def maxBear = Max(hang, Max(sstar, Max(sEng, Max(fall3, Max(crow, Max(estar, Max(sHar, TwzT)))))));
def hiBull = if maxBull == ham then 8 else
if maxBull == inv then 7 else
if maxBull == bEng then 6 else
if maxBull == rsi3 then 5 else
if maxBull == sold then 4 else
if maxBull == mstar then 3 else
if maxBull == bHar then 2 else 1;
def hiBear = if maxBear == hang then 8 else
if maxBear == sstar then 7 else
if maxBear == sEng then 6 else
if maxBear == fall3 then 5 else
if maxBear == crow then 4 else
if maxBear == estar then 3 else
if maxBear == sHar then 2 else 1;
AddLabel(top and maxBull and hiBull==8, "Hammer(" + hammer_per + "%)", Color.CYAN);
AddLabel(top and maxBull and hiBull==7, "Inverted Hammer(" + inv_hammer_per + "%)", Color.CYAN);
AddLabel(top and maxBull and hiBull==6, "Bullish Engulfing(" + bull_engulfing_per + "%)", Color.CYAN);
AddLabel(top and maxBull and hiBull==5, "Rising 3(" + rising_3_per + "%)", Color.CYAN);
AddLabel(top and maxBull and hiBull==4, "3 White Soldiers(" + soldiers_per + "%)", Color.CYAN);
AddLabel(top and maxBull and hiBull==3, "Morning Star(" + m_star_per + "%)", Color.CYAN);
AddLabel(top and maxBull and hiBull==2, "Bull Harami(" + bull_harami_per + "%)", Color.CYAN);
AddLabel(top and maxBull and hiBull==1, "Tweezer Bottom(" + tweezer_btm_per + "%)", Color.CYAN);
# bear
AddLabel(top and maxBear and hiBear==8, "Hanging Man(" + h_man_per + "%)", Color.MAGENTA);
AddLabel(top and maxBear and hiBear==7, "Shooting Star(" + s_star_per + "%)", Color.MAGENTA);
AddLabel(top and maxBear and hiBear==6, "Bear Engulfing(" + bear_engulfing_per + "%)", Color.MAGENTA);
AddLabel(top and maxBear and hiBear==5, "Falling 3(" + falling_3_per + "%)", Color.MAGENTA);
AddLabel(top and maxBear and hiBear==4, "3 Black Crows(" + crows_per + "%)", Color.MAGENTA);
AddLabel(top and maxBear and hiBear==3, "Evening Star(" + e_star_per + "%)", Color.MAGENTA);
AddLabel(top and maxBear and hiBear==2, "Bear Harami(" + bear_harami_per + "%)", Color.MAGENTA);
AddLabel(top and maxBear and hiBear==1, "Tweezer Top(" + tweezer_top_per + "%)", Color.MAGENTA);
#-- end of code