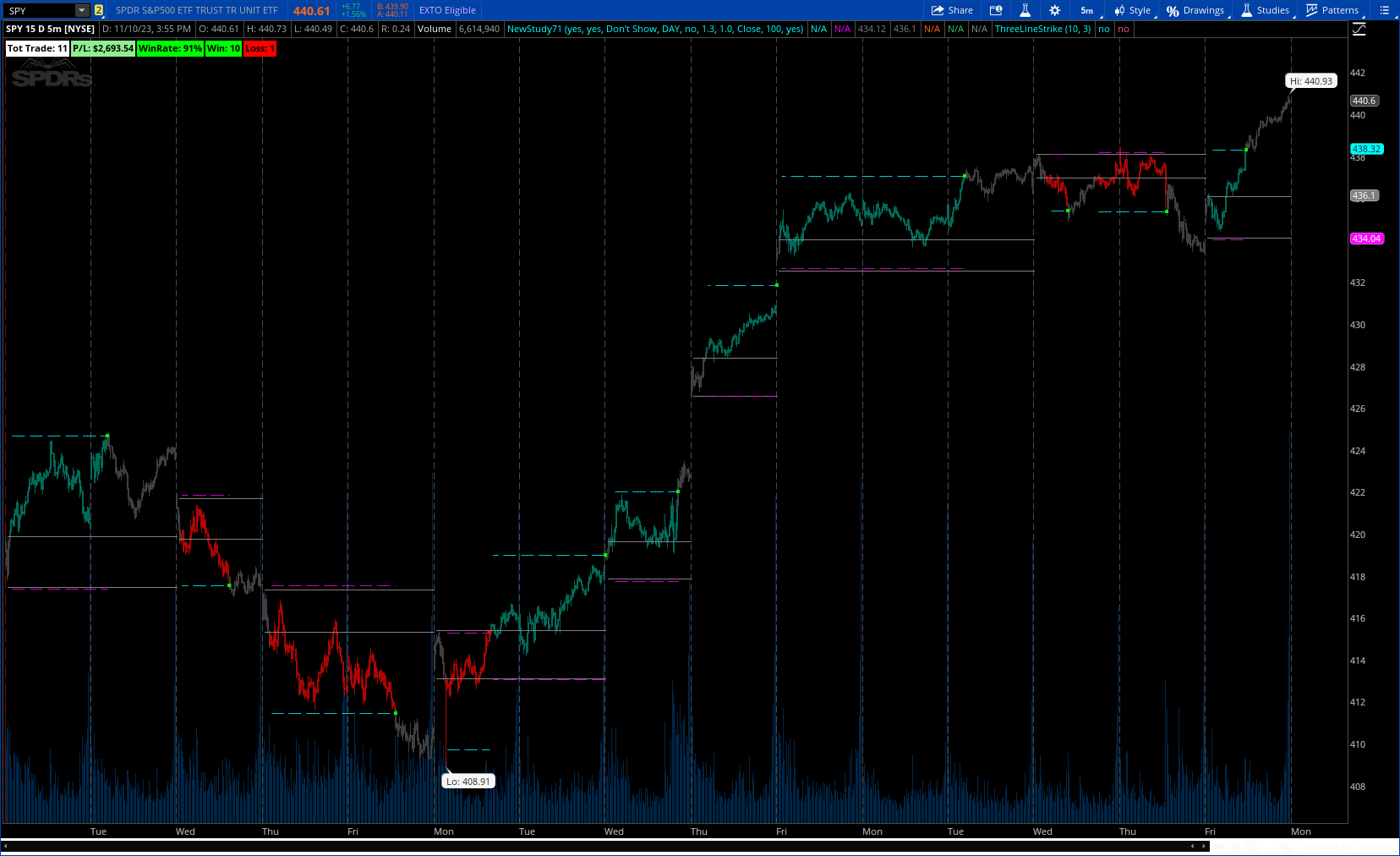
Author Message:
The Ranges With Targets indicator is a tool designed to assist traders in identifying potential trading opportunities on a chart derived from breakout trading. It dynamically outlines ranges with boxes in real-time, providing a visual representation of price movements. When a breakout occurs from a range, the indicator will begin coloring the candles. A green candle signals a long breakout, suggesting a potential upward movement, while a red candle indicates a short breakout, suggesting a potential downward movement. Grey candles indicate periods with no active trade. Ranges are derived from daily changes in price action.
CODE:
CSS:
# https://www.tradingview.com/v/3swrxpw6/
#// This source code is subject to the terms of the Mozilla Public License 2.0
#// © ChartPrime
#indicator("Ranges with Targets [ChartPrime]",overlay = true,max_lines_count = 500,max_labels_count = 500)
# converted by Sam4Cok@Samer800 - 11/2023
input colorBars = yes;
input showInfoLabel = yes;
input signalType = {default "Bubbles", "Arrows", "Don't Show"};
input rangeTimeFrame = {default DAY, WEEK, MONTH};
input atrBodyFilter = no; # "ATR Body Filter"
input profitMulti = 1.3; # "Target Multiplyer"
input stoplossMulti = 1.0; # "stop Multiplyer"
input stoplossType = {default "Close", "High/Low"}; # 'SL Type'
input lotSizeinUsd = 100;#,"Risk Per Trade (USD)",group=CORE)
input showTargetLines = yes;
def na = Double.NaN;
def bar_index = AbsValue(BarNumber());
def sigBubble = signalType == signalType."Bubbles";
def sigArrows = signalType == signalType."Arrows";
def slClose = stoplossType == stoplossType."Close";
DefineGlobalColor("Line", Color.GRAY);#CreateColor(245,127,23));#CreateColor(92, 67, 154));
DefineGlobalColor("RED", CreateColor(172, 5, 5));
DefineGlobalColor("GREEN", CreateColor(2, 106, 89));
DefineGlobalColor("OFF", Color.DARK_GRAY);#CreateColor(52, 52, 54));
def minVol = Min(ATR(Length = 30) * 0.3, close * (0.3 / 100));
def volAdj = minVol[20] / 2;
def BodyRange = AbsValue(close - open);
def BodyCon = bar_index > 0;
def BodyCon1 = (if atrBodyFilter then BodyRange < ATR(Length = 5) else yes);
script VolCal {
input Index = 100;
def var = AbsValue(Index);
def Bar = Max(Min(var, 500), 1);
def Bars = if IsNaN(Bar) then 1 else Bar;
def Green = fold i = 0 to Bars with p do
if GetValue(close, i) > GetValue(open, i) then p + GetValue(volume, i) else p;
def Red = fold j = 0 to Bars with q do
if GetValue(close, j) < GetValue(open, j) then q + GetValue(volume, j) else q;
def Total = fold v = 0 to Bars with l do
l + GetValue(volume, v);
def GreenRatio = Green / Total * 100;
def RedRatio = Red / Total * 100;
def VolCal = if GreenRatio > 55 then 1 else
if RedRatio > 55 then -1 else 0;
plot Out = VolCal;
}
def nATR;
def SCR;
def TradeOn;
def Res;
def TradeisON;
def yyyyMmDd = GetYYYYMMDD();
def periodIndx;
switch (rangeTimeFrame) {
case DAY:
periodIndx = yyyyMmDd;
case WEEK:
periodIndx = Floor((DaysFromDate(First(yyyyMmDd)) + GetDayOfWeek(First(yyyyMmDd))) / 7);
case MONTH:
periodIndx = RoundDown(yyyyMmDd / 100, 0);
}
def isPeriodRolled = CompoundValue(1, periodIndx != periodIndx[1], yes);
if isPeriodRolled[1] and !TradeisON[1] {
nATR = volAdj;
SCR = hl2 - (nATR * 15);
Res = SCR + (nATR * 25);
TradeOn = yes;
} else {
nATR = 0;
SCR = SCR[1];
Res = Res[1];
TradeOn = no;
}
def crossOver = Crosses(close, Res, CrossingDirection.ABOVE);
def crossUnder = Crosses(close, SCR, CrossingDirection.BELOW);
def BUY = crossOver[1] and BodyCon and BodyCon1;
def SELL = crossUnder[1] and BodyCon and BodyCon1;
#//----- { SL Calculation
def LongTrade;
def ShortTrade;
def longDiffSL2 = AbsValue(close - Res);
def longDiffSL = AbsValue(close - SCR);
def Long = BUY and !TradeisON[1];
def Short = SELL and !TradeisON[1];
def TradeFire = Long or Short;
if Long and !TradeisON[1] {
LongTrade = yes;
ShortTrade = no;
} else
if Short and !TradeisON[1] {
LongTrade = no;
ShortTrade = yes;
} else {
LongTrade = LongTrade[1];
ShortTrade = ShortTrade[1];
}
def win;
def los;
def entry;
def profit;
def losses;
def TP;
def SL;
if TradeFire and !TradeisON[1] {
entry = ohlc4[-1];
TP = if Long then entry + (profitMulti * longDiffSL) else
if Short then entry - (profitMulti * longDiffSL2) else na;
SL = if Long then entry - (stoplossMulti * longDiffSL) else
if Short then entry + (stoplossMulti * longDiffSL2) else na;
win = if !bar_index then 0 else win[1];
los = if !bar_index then 0 else los[1];
profit = profit[1];
losses = losses[1];
TradeisON = yes;
} else
if LongTrade and TradeisON[1] {
entry = entry[1];
TP = TP[1];
SL = SL[1];
win = if high >= TP then win[1] + 1 else win[1];
los = if close <= SL then los[1] + 1 else los[1];
profit = if (high crosses above TP) then profit[1] + AbsValue(close - entry) * lotSizeinUsd else profit[1];
losses = if (close crosses below SL) then losses[1] + AbsValue(entry - close) * lotSizeinUsd else losses[1];
TradeisON = if high >= TP then no else
if (if slClose then close else low) <= SL then no else TradeisON[1];
} else
if ShortTrade and TradeisON[1] {
entry = entry[1];
TP = TP[1];
SL = SL[1];
win = if low <= TP then win[1] + 1 else win[1];
los = if close >= SL then los[1] + 1 else los[1];
profit = if (low crosses below TP) then profit[1] + AbsValue(entry - close) * lotSizeinUsd else profit[1];
losses = if (close crosses above SL) then losses[1] + AbsValue(close - entry) * lotSizeinUsd else losses[1];
TradeisON = if low <= TP then no else
if (if slClose then close else high) >= SL then no else TradeisON[1];
} else {
entry = na;
win = if !bar_index then 0 else win[1];
los = if !bar_index then 0 else los[1];
profit = profit[1];
losses = losses[1];
TradeisON = TradeisON[1];
TP = if TradeisON then TP[1] else na;
SL = if TradeisON then SL[1] else na;
}
def lineUp = !showTargetLines or TP != TP[1];
def lineDn = !showTargetLines or SL != SL[1];
plot TakeProfit = if lineUp then na else TP;
plot stopLoss = if lineDn then na else SL;
TakeProfit.SetStyle(Curve.MEDIUM_DASH);
stopLoss.SetStyle(Curve.MEDIUM_DASH);
TakeProfit.SetDefaultColor(Color.CYAN);
stopLoss.SetDefaultColor(Color.MAGENTA);
plot supp = if !TradeOn and SCR then SCR else na;
plot ress = if !TradeOn and Res then Res else na;
#supp.SetPaintingStrategy(PaintingStrategy.HORIZONTAL);
#ress.SetPaintingStrategy(PaintingStrategy.HORIZONTAL);
supp.SetDefaultColor(GlobalColor("Line"));
ress.SetDefaultColor(GlobalColor("Line"));
#--BarColor
def BearCon = TradeisON and ShortTrade;
def BullCon = TradeisON and LongTrade;
AssignPriceColor(if !colorBars then Color.CURRENT else
if BearCon then GlobalColor("RED") else
if BullCon then GlobalColor("GREEN") else GlobalColor("OFF"));
#-- Backtest
plot TradeEnd = if !TradeisON and TradeisON[1] then
if los > los[1] then SL[1] else TP[1] else na;
TradeEnd.AssignValueColor(if win > win[1] then Color.GREEN else Color.RED);
TradeEnd.SetPaintingStrategy(PaintingStrategy.SQUARES);
#-- Label
def totTrade = win + los;
def winRate = Round(win / totTrade * 100, 0);
def PandL = Round(profit - losses, 2);
AddLabel(showInfoLabel, "Tot Trade: " + totTrade, Color.WHITE);
AddLabel(showInfoLabel, "P/L: $" + PandL,
if PandL > 0 then Color.LIGHT_GREEN else
if PandL < 0 then Color.PINK else Color.GRAY);
AddLabel(showInfoLabel, "WinRate: " + winRate + "%",
if winRate > 50 then Color.GREEN else
if winRate < 50 then Color.RED else Color.GRAY);
AddLabel(showInfoLabel, "Win: " + win, Color.GREEN);
AddLabel(showInfoLabel, "Loss: " + los, Color.RED);
#-- Signals
def volH = VolCal(30);
def volL = VolCal(30);
def signH = if Long then volH else signH[1];
def signS = if Short then volL else signS[1];
def buyCond = BullCon and !BullCon[1];
def sellCond = BearCon and !BearCon[1];
#-- Signals
plot arrUp = if sigArrows and buyCond then low - minVol else na;
plot arrDn = if sigArrows and sellCond then high + minVol else na;
arrUp.SetPaintingStrategy(PaintingStrategy.ARROW_UP);
arrDn.SetPaintingStrategy(PaintingStrategy.ARROW_DOWN);
arrUp.AssignValueColor(if signH > 0 then Color.CYAN else Color.VIOLET);
arrDn.AssignValueColor(if signS < 0 then Color.MAGENTA else Color.PLUM);
AddChartBubble(sigBubble and buyCond, low, "B" , if signH > 0 then Color.GREEN else Color.DARK_GREEN, no);
AddChartBubble(sigBubble and sellCond, high, "S", if signS < 0 then Color.RED else Color.DARK_RED);
#-- END of CODE