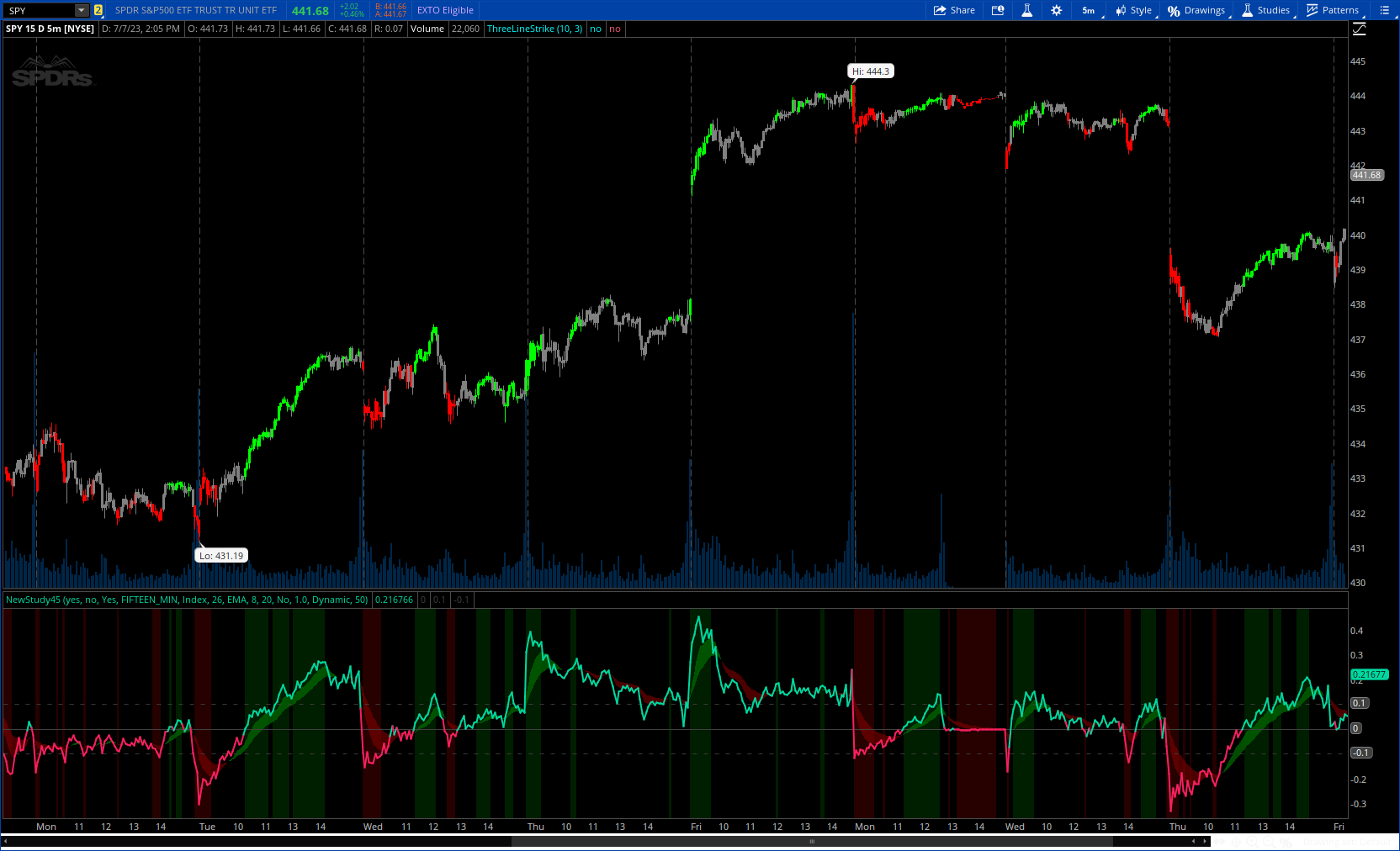
Author Message:
Dynamic Money Flow is a volume indicator based on Marc Chaikin's Money Flow with a few improvements.
It can be used to confirm break-outs and trends.
Zero line crosses and divergences can provide useful signals while considering chart analysis as well.
More details : https://www.tradingview.com/v/c7z3p2xh/
CODE:
CSS:
# https://www.tradingview.com/v/c7z3p2xh/
#// © RezzaHmt
#//...DMF...
#study("Dynamic Money Flow", "DMF", format=format.volume, precision=2, resolution="")
#-- Converted and mod by Sam4Cok@Samer800 - 07/07/2023
declare lower;
input colorBars = yes;
input showCloud = no;
input useChartTimeframe = {default"Yes", "No"};
input manualTimeframe = AggregationPeriod.FIFTEEN_MIN;
input mode = {default "Index", "Cumulative"};
input indexModePeriod = 26; # "Period"-"Only applies to index mode."
input ma_switch = {"OFF", default "EMA", "WMA"}; # "Moving Averages"
input fast_len = 8; # "Fast Length"
input slow_len = 20; # "Slow Length"
input simulative_vol = {"Yes", default "No"}; # "Simulative Volume"
input volumePower = 1.0; # "Power"
input weight_distribution = {default "Dynamic", "Static"};# "Weight Distribution Method"
input static_Distribution_Per = 50;# "Static Weight Distribution Bias"
def na = Double.NaN;
def last = isNaN(close);
def pos = Double.POSITIVE_INFINITY;
def neg = Double.NEGATIVE_INFINITY;
def index = mode == mode."Index";
def noMA = ma_switch==ma_switch."OFF";
#-- Color.
DefineGlobalColor("up", CreateColor(0,216,160));
DefineGlobalColor("dn", CreateColor(248,32,96));
#-- MTF
def c; def h; def l; def o; def v;
Switch (useChartTimeframe) {
case "Yes" :
c = close;
h = high;
l = low;
o = open;
v = volume;
case "No" :
c = close(Period=manualTimeframe);
h = high(Period=manualTimeframe);
l = low(Period=manualTimeframe);
o = open(Period=manualTimeframe);
v = volume(Period=manualTimeframe);
}
def AbsClose = AbsValue(c - c[1]);
def AbsHigh = AbsValue(h - Max(o, c));
def AbsOpen = AbsValue(Min(o, c) - l);
def vol;
switch (simulative_vol) {
case "Yes" :
vol = Power(AbsClose + AbsHigh * 2 + AbsOpen * 2, volumePower);
case "No" :
vol = Power(v, volumePower);
}
def tr = TrueRange(h, c, l);
def alpha;
switch (weight_distribution) {
case "Dynamic" :
alpha = if tr == 0 then 0 else AbsValue((c - c[1]) / tr);
case "Static" :
alpha = static_Distribution_Per / 100;
}
def trh = Max(h, c[1]);
def trl = Min(l , c[1]);
def ctr = if tr == 0 then 0 else ((c - trl) + (c - trh)) / tr * (1 - alpha) * vol;
def ctc = if c > c[1] then alpha * vol else
if c < c[1] then -alpha * vol else 0;
def ctrSum = ctrSum[1] + ctr;
def ctcSum = ctcSum[1] + ctc;
def ctSum = ctr + ctc;
def dmfCT = WildersAverage(ctSum, indexModePeriod);
def dmfVo = WildersAverage(vol, indexModePeriod);
def dmf;
switch (mode) {
case "Index" :
dmf = dmfCT / dmfVo;
case "Cumulative" :
dmf = ctrSum + ctcSum;
}
def fast_ma;
switch (ma_switch) {
case "OFF" :
fast_ma = na;
case "EMA" :
fast_ma = ExpAverage(dmf, fast_len);
case "WMA" :
fast_ma = WMA(dmf, fast_len);
}
def slow_ma;
switch (ma_switch) {
case "OFF" :
slow_ma = na;
case "EMA" :
slow_ma = ExpAverage(dmf, slow_len);
case "WMA" :
slow_ma = WMA(dmf, slow_len);
}
def main_color;
switch (mode) {
case "Index" :
main_color = if dmf > 0 then 1 else if dmf < 0 then -1 else 1;
case "Cumulative" :
main_color = if slow_ma and dmf > slow_ma then 1 else
if slow_ma and dmf < slow_ma then -1 else 1;
}
plot pMain = dmf; # "DMF"
def pZero = if Index then 0 else na;
def pFastMA = fast_ma; # "Fast MA"
def pSlowMA = slow_ma;
pMain.SetLineWeight(2);
pMain.AssignValueColor(if main_color>0 then GlobalColor("up") else
if main_color<0 then GlobalColor("dn") else GlobalColor("up"));
AddCloud(if !showCloud then na else pMain, pZero, GlobalColor("up"), GlobalColor("dn"));#"Oscillator Background"
AddCloud(pFastMA, pSlowMA, Color.GREEN, Color.RED, showCloud);# "Moving Average Fill"
plot "0" = if Index and !last then 0 else na;#, "Zero Line"
plot "1" = if Index and !last then 0.1 else na;#, "Level"
plot "-1" = if Index and !last then -0.1 else na;#, "Level"
"1".SetDefaultColor(Color.DARK_GRAY);
"0".SetDefaultColor(Color.DARK_GRAY);
"-1".SetDefaultColor(Color.DARK_GRAY);
"1".SetStyle(Curve.SHORT_DASH);
"-1".SetStyle(Curve.SHORT_DASH);
#-- Background
def upBG = if noMA then main_color > -0.1 and dmf > wma(dmf, 10) else
dmf>pFastMA and main_color>-0.1 and pFastMA>pSlowMA;
def dnBG = if noMA then main_color < 0.1 and dmf < wma(dmf, 10) else
dmf<pFastMA and main_color<0.1 and pFastMA<pSlowMA;
AddCloud(if upBG then pos else na, neg, Color.DARK_GREEN);
AddCloud(if dnBG then pos else na, neg, Color.DARK_RED);
#-- Bar Color
AssignPriceColor(if !colorBars then Color.CURRENT else
if upBG then Color.GREEN else
if dnBG then COlor.RED else Color.GRAY);
#--- END of CODE