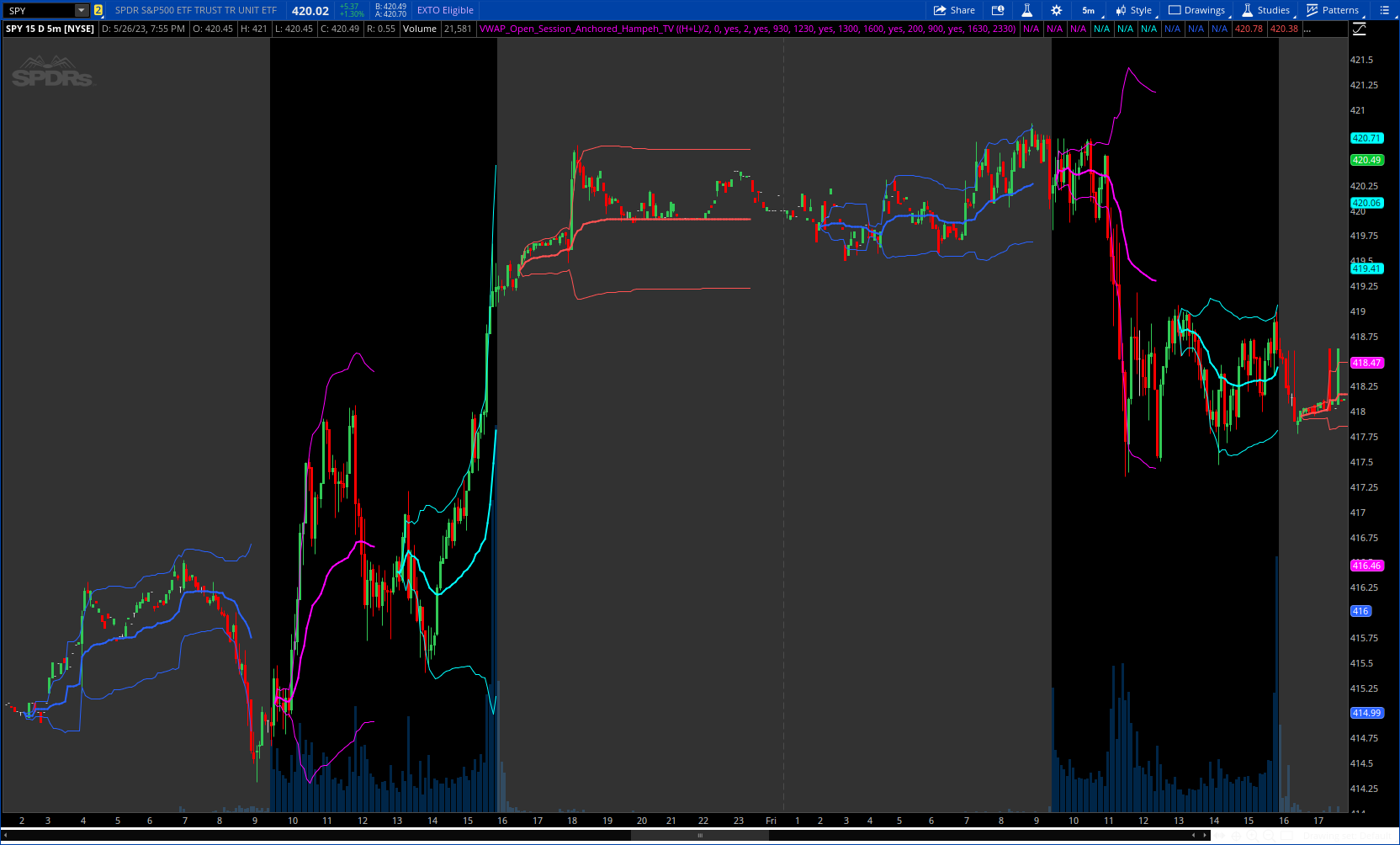
The VWAP Open Session Anchored indicator differs from traditional VWAP indicators by automatically anchoring the Volume Weighted Average Price calculation to three market session starts Morning, Evening, and Night. Each session represents a distinct time period within the trading day, offering traders and investors a more comprehensive view of the volume-weighted average price within specific sessions.
CODE:
CSS:
#// This source code is subject to the terms of the Mozilla Public License 2.0 at https://mozilla.org/MPL/2.0/
# @Hampeh
#indicator("VWAP Open Session Anchored by Hampeh", "VWAP OPen Session", true)
# Converted and mod by Sam4Cok@Samer800 - 05/2023
input source = hl2; # "Source"
input offset = 0; # "Offset"
input showBands = no; # "Band Multiplier"
input multiplier = 2; # "Multiplier"
input morning = yes;
input morningSessionStartTime = 0930;
input morningSessionEndTime = 1230;
input evening = yes;
input eveningSessionStartTime = 1400;
input eveningSessionEndTime = 1600;
input preMarket = yes;
input preMarketStartTime = 0200;
input preMarketEndTime = 0900;
input afterHours = yes;
input afterHoursStartTime = 1630;
input afterHoursEndTime = 2330;
def na = Double.NaN;
def morningSession = SecondsTillTime(morningSessionStartTime) < 0 and SecondsTillTime(morningSessionEndTime) > 0;
def eveningSession = SecondsTillTime(eveningSessionStartTime) < 0 and SecondsTillTime(eveningSessionEndTime) > 0;
def premarketSession = SecondsTillTime(preMarketStartTime) < 0 and SecondsTillTime(preMarketEndTime) > 0;
def afterHoursSession = SecondsTillTime(afterHoursStartTime) < 0 and SecondsTillTime(afterHoursEndTime) > 0;
#--- Color
DefineGlobalColor("morining" , Color.MAGENTA);
DefineGlobalColor("evening" , Color.CYAN);
DefineGlobalColor("premarket" , CreateColor(41,98,255));
DefineGlobalColor("afterHours" , CreateColor(255,82,82));
#export f_Vwap(simple string tf, float src, float src_v) =>
script f_Vwap {
input src = close;
input tf = close;
input multiplier = 1;
def src_v = volume;
def start0 = tf - tf[1];
def sumSrc0 = src * src_v;
def sumVol0 = src_v;
def sumSrc2 = src_v * Sqr(src);
def sumSrc1 = CompoundValue(1, if start0 then sumSrc0 else sumSrc0 + sumSrc1[1], sumSrc0);
def sumVol1 = CompoundValue(1, if start0 then sumVol0 else sumVol0 + sumVol1[1], sumVol0);
def sumVol2 = CompoundValue(1, if start0 then sumSrc2 else sumSrc2 + sumVol2[1], sumSrc2);
def Vwap = sumSrc1 / sumVol1;
def deviation = Sqrt(Max(sumVol2 / sumVol1 - Sqr(Vwap), 0));
plot wap = Vwap;
plot Upper = Vwap + deviation * multiplier;
plot Lower = Vwap - deviation * multiplier;
}
def morningVwap = f_Vwap(source, morningSession, multiplier).wap;
def morningUpper = f_Vwap(source, morningSession, multiplier).Upper;
def morningLower = f_Vwap(source, morningSession, multiplier).Lower;
def eveningVwap = f_Vwap(source, eveningSession, multiplier).wap;
def eveningUpper = f_Vwap(source, eveningSession, multiplier).Upper;
def eveningLower = f_Vwap(source, eveningSession, multiplier).Lower;
def premarketVwap = f_Vwap(source, premarketSession, multiplier).wap;
def premarketUpper = f_Vwap(source, premarketSession, multiplier).Upper;
def premarketLower = f_Vwap(source, premarketSession, multiplier).Lower;
def nightVwap = f_Vwap(source, afterHoursSession, multiplier).wap;
def nightUpper = f_Vwap(source, afterHoursSession, multiplier).Upper;
def nightLower = f_Vwap(source, afterHoursSession, multiplier).Lower;
#// PLOTS
plot plotMorningUpper = if morning and showBands and morningSession then morningUpper[offset] else na;
plotMorningUpper.SetDefaultColor(GlobalColor("morining"));
plot plotMorningVwap = if morning and morningSession then morningVwap[offset] else na;
plotMorningVwap.SetDefaultColor(GlobalColor("morining"));
plotMorningVwap.SetLineWeight(2);
plot plotMorningLower = if morning and showBands and morningSession then morningLower[offset] else na;
plotMorningLower.SetDefaultColor(GlobalColor("morining"));
plot plotEveningUpper = if evening and showBands and eveningSession then eveningUpper[offset] else na;
plotEveningUpper.SetDefaultColor(GlobalColor("evening"));
plot plotEveningVwap = if evening and eveningSession then eveningVwap[offset] else na;
plotEveningVwap.SetDefaultColor(GlobalColor("evening"));
plotEveningVwap.SetLineWeight(2);
plot plotEveningLower = if evening and showBands and eveningSession then eveningLower[offset] else na;
plotEveningLower.SetDefaultColor(GlobalColor("evening"));
plot plotPremarketUpper = if preMarket and showBands and premarketSession then premarketUpper[offset] else na;
plotPremarketUpper.SetDefaultColor(GlobalColor("premarket"));
plot plotPremarketVwap = if preMarket and premarketSession then premarketVwap[offset] else na;
plotPremarketVwap.SetDefaultColor(GlobalColor("premarket"));
plotPremarketVwap.SetLineWeight(2);
plot plotPremarketLower = if preMarket and showBands and premarketSession then premarketLower[offset] else na;
plotPremarketLower.SetDefaultColor(GlobalColor("premarket"));
plot plotAfterHoursNightUpper = if afterHours and showBands and afterHoursSession then nightUpper[offset] else na;
plotAfterHoursNightUpper.SetDefaultColor(GlobalColor("afterHours"));
plot plotAfterHoursVwap = if afterHours and afterHoursSession then nightVwap[offset] else na;
plotAfterHoursVwap.SetDefaultColor(GlobalColor("afterHours"));
plotAfterHoursVwap.SetLineWeight(2);
plot plotAfterHoursLower = if afterHours and showBands and afterHoursSession then nightLower[offset] else na;
plotAfterHoursLower.SetDefaultColor(GlobalColor("afterHours"));
#-- END of Code