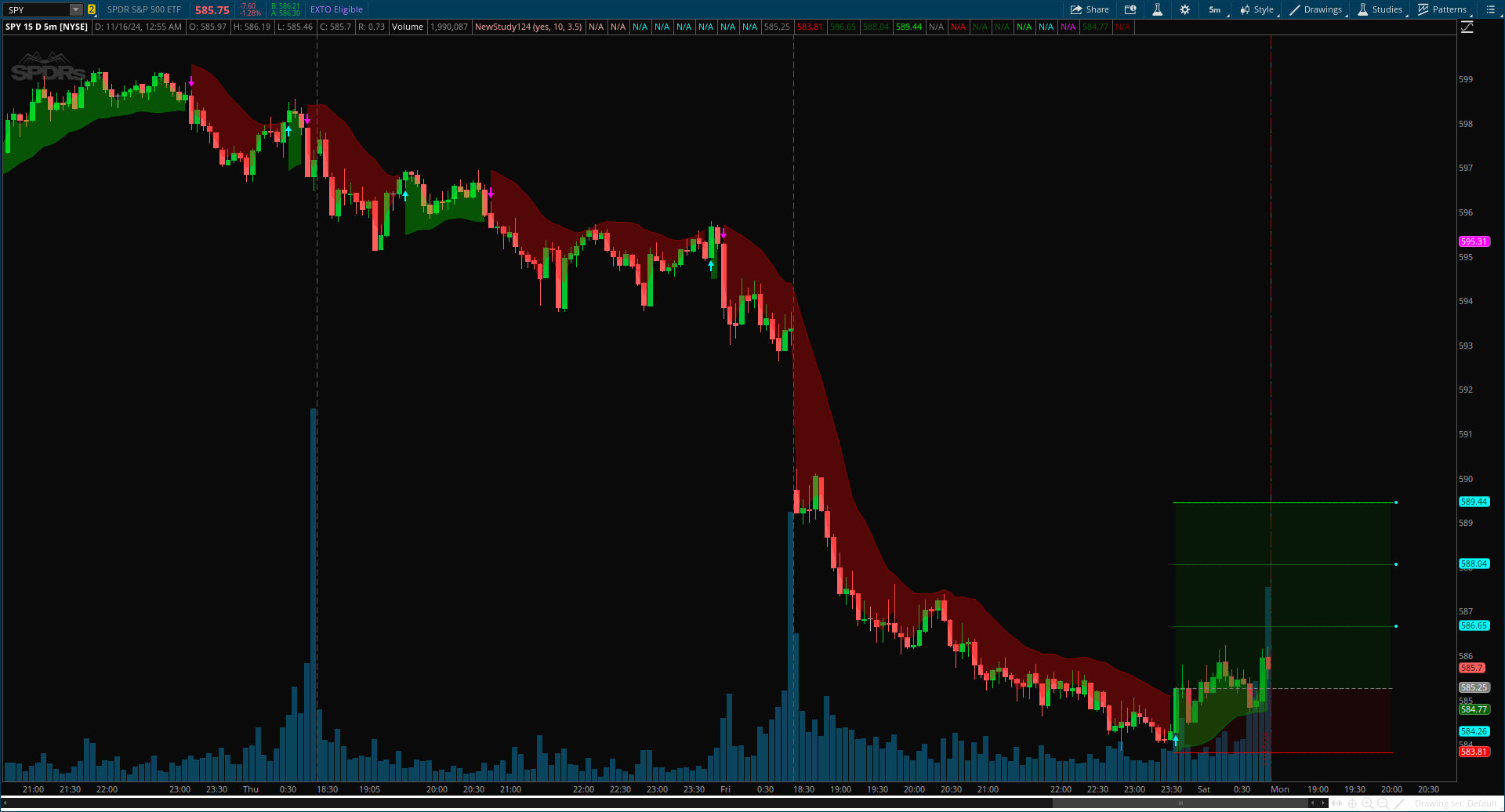
Author Message:
The Target Trend indicator is a trend-following tool designed to assist traders in capturing directional moves while managing entry, stop loss, and profit targets visually on the chart. Using adaptive SMA bands as the core trend detection method, this indicator dynamically identifies shifts in trend direction and provides structured exit points through customizable target levels.
CODE:
CSS:
#// Indicator for TOS
#// © BigBeluga
#indicator("Target Trend [BigBeluga]", overlay = true, max_lines_count = 40)
input showLastTrendOnly = yes;
input length = 10; #, "Trend Length")
input targetMultiplier = 3.5; #, "Set Targets")
def na = Double.NaN;
def last = IsNaN(close);
def bar = BarNumber();
def hi = if !last then high else hi[1];
def lo = if !last then low else lo[1];
#-- Color
DefineGlobalColor("up", CreateColor(0, 57, 0));
DefineGlobalColor("dn", CreateColor(67, 0, 0));
#// ATR for calculating stop loss and target levels
def nATR = ATR(Length = 200);
def atr_value = Average(nATR, 200) * 0.8;
#// Moving averages for trend detection
def smaHi = Average(high, length);
def smaLo = Average(low, length);
def sma_high = smaHi + atr_value;
def sma_low = smaLo - atr_value;
def crossUp = (close > sma_high) and (close[1] <= sma_high[1]) and !last;
def crossDn = (close < sma_low) and (close[1] >= sma_low[1]) and !last;
def trend1 = if crossUp then yes else if crossDn then no else trend1[1];
def trend = if !isNaN(trend1) then trend1 else trend[1];
def cntUp = if trend then cntUp[1] + 1 else if !trend then 0 else cntUp[1];
def cntDn = if !trend then cntDn[1] + 1 else if trend then 0 else cntDn[1];
#/ Signal detection for trend changes
def signal_up = (trend - trend[1]) and !trend[1];
def signal_dn = (trend - trend[1]) and trend[1];
def cnt = if signal_up then bar else if signal_dn then bar else cnt[1];
def cond = if showLastTrendOnly then bar >= highestAll(cnt) else yes;
#-- Targets
def baseUp;
def stopUp;
def tgtUp1; def tgtUp2; def tgtUp3;
if signal_up and cond{
baseUp = close;
stopUp = sma_low;
tgtUp1 = close + atr_value * (targetMultiplier * 1);
tgtUp2 = close + atr_value * (targetMultiplier * 2);
tgtUp3 = close + atr_value * (targetMultiplier * 3);
} else if signal_dn {
baseUp = na;
stopUp = na;
tgtUp1 = na;
tgtUp2 = na;
tgtUp3 = na;
} else {
tgtUp1 = if ((hi[1] >= tgtUp1[1] and cntUp > 1) or last[20]) then na else tgtUp1[1];
tgtUp2 = if ((hi[1] >= tgtUp2[1] and cntUp > 1) or last[20]) then na else tgtUp2[1];
tgtUp3 = if ((hi[1] >= tgtUp3[1] and cntUp > 1) or last[20]) then na else tgtUp3[1];
baseUp = if isNaN(tgtUp3) then na else baseUp[1];
stopUp = if isNaN(tgtUp3) then na else stopUp[1];
}
def baseDn;
def stopDn;
def tgtDn1; def tgtDn2; def tgtDn3;
if signal_dn and cond{
baseDn = close;
stopDn = sma_high;
tgtDn1 = close - atr_value * (targetMultiplier * 1);
tgtDn2 = close - atr_value * (targetMultiplier * 2);
tgtDn3 = close - atr_value * (targetMultiplier * 3);
} else if signal_up {
baseDn = na;
stopDn = na;
tgtDn1 = na;
tgtDn2 = na;
tgtDn3 = na;
} else {
tgtDn1 = if ((lo[1] <= tgtDn1[1] and cntDn > 1) or last[20]) then na else tgtDn1[1];
tgtDn2 = if ((lo[1] <= tgtDn2[1] and cntDn > 1) or last[20]) then na else tgtDn2[1];
tgtDn3 = if ((lo[1] <= tgtDn3[1] and cntDn > 1) or last[20]) then na else tgtDn3[1];
baseDn = if isNaN(tgtDn3) then na else baseDn[1];
stopDn = if isNaN(tgtDn3) then na else stopDn[1];
}
plot lostLong = if (signal_dn and (close <= stopUp[1])) then stopUp[1] else na;
plot lostShort = if (signal_up and (close >= stopDn[1])) then stopDn[1] else na;
plot tgtPtUp1 = if (isNaN(tgtUp1) and tgtUp1[1] and !signal_dn) then tgtUp1[1] else na;
plot tgtPtUp2 = if (isNaN(tgtUp2) and tgtUp2[1] and !signal_dn) then tgtUp2[1] else na;
plot tgtPtUp3 = if (isNaN(tgtUp3) and tgtUp3[1] and !signal_dn) then tgtUp3[1] else na;
plot tgtPtDn1 = if (isNaN(tgtDn1) and tgtDn1[1] and !signal_up) then tgtDn1[1] else na;
plot tgtPtDn2 = if (isNaN(tgtDn2) and tgtDn2[1] and !signal_up) then tgtDn2[1] else na;
plot tgtPtDn3 = if (isNaN(tgtDn3) and tgtDn3[1] and !signal_up) then tgtDn3[1] else na;
plot entryLong = if baseUp then baseUp else na;
plot slLong = if stopUp then stopUp else na;
plot tgtLong1 = if tgtUp1 then tgtUp1 else na;
plot tgtLong2 = if tgtUp2 then tgtUp2 else na;
plot tgtLong3 = if tgtUp3 then tgtUp3 else na;
plot entryShort = if baseDn then baseDn else na;
plot slShort = if stopDn then stopDn else na;
plot tgtShort1 = if tgtDn1 then tgtDn1 else na;
plot tgtShort2 = if tgtDn2 then tgtDn2 else na;
plot tgtShort3 = if tgtDn3 then tgtDn3 else na;
lostLong.SetDefaultColor(Color.PINK);
lostShort.SetDefaultColor(Color.PINK);
lostLong.SetPaintingStrategy(PaintingStrategy.SQUARES);
lostShort.SetPaintingStrategy(PaintingStrategy.SQUARES);
tgtPtUp1.SetDefaultColor(Color.CYAN);
tgtPtUp2.SetDefaultColor(Color.CYAN);
tgtPtUp3.SetDefaultColor(Color.CYAN);
tgtPtUp1.SetPaintingStrategy(PaintingStrategy.POINTS);
tgtPtUp2.SetPaintingStrategy(PaintingStrategy.POINTS);
tgtPtUp3.SetPaintingStrategy(PaintingStrategy.POINTS);
entryLong.SetDefaultColor(Color.GRAY);
slLong.SetDefaultColor(Color.RED);
tgtLong1.SetDefaultColor(Color.DARK_GREEN);
tgtLong2.SetDefaultColor(Color.DARK_GREEN);
tgtLong3.SetDefaultColor(Color.GREEN);
entryLong.SetPaintingStrategy(PaintingStrategy.DASHES);
slLong.SetPaintingStrategy(PaintingStrategy.HORIZONTAL);
tgtLong1.SetPaintingStrategy(PaintingStrategy.HORIZONTAL);
tgtLong2.SetPaintingStrategy(PaintingStrategy.HORIZONTAL);
tgtLong3.SetPaintingStrategy(PaintingStrategy.HORIZONTAL);
tgtPtDn1.SetDefaultColor(Color.CYAN);
tgtPtDn2.SetDefaultColor(Color.CYAN);
tgtPtDn3.SetDefaultColor(Color.CYAN);
tgtPtDn1.SetPaintingStrategy(PaintingStrategy.POINTS);
tgtPtDn2.SetPaintingStrategy(PaintingStrategy.POINTS);
tgtPtDn3.SetPaintingStrategy(PaintingStrategy.POINTS);
entryShort.SetDefaultColor(Color.GRAY);
slShort.SetDefaultColor(Color.RED);
tgtShort1.SetDefaultColor(Color.DARK_GREEN);
tgtShort2.SetDefaultColor(Color.DARK_GREEN);
tgtShort3.SetDefaultColor(Color.GREEN);
entryShort.SetPaintingStrategy(PaintingStrategy.DASHES);
slShort.SetPaintingStrategy(PaintingStrategy.HORIZONTAL);
tgtShort1.SetPaintingStrategy(PaintingStrategy.HORIZONTAL);
tgtShort2.SetPaintingStrategy(PaintingStrategy.HORIZONTAL);
tgtShort3.SetPaintingStrategy(PaintingStrategy.HORIZONTAL);
#// Plot trailing stops
def p0 = hl2;
plot sigUp = if signal_up then low else na;
plot sigDn = if signal_dn then high else na;
plot trendUp = if trend then sma_low else na;
plot trendDn = if !trend then sma_high else na;
sigUp.SetDefaultColor(Color.CYAN);
sigDn.SetDefaultColor(Color.MAGENTA);
sigUp.SetPaintingStrategy(PaintingStrategy.BOOLEAN_ARROW_UP);
sigDn.SetPaintingStrategy(PaintingStrategy.BOOLEAN_ARROW_DOWN);
trendUp.SetDefaultColor(Color.DARK_GREEN);
trendDn.SetDefaultColor(Color.DARK_RED);
AddCloud(p0, trendUp, Color.GREEN);
AddCloud(trendDn, p0, Color.RED);
AddCloud(EntryLong, slLong, GlobalColor("dn"));
AddCloud(slShort, entryShort, GlobalColor("dn"));
AddCloud(tgtLong3, EntryLong, GlobalColor("up"));
AddCloud(entryShort, tgtShort3, GlobalColor("up"));
#-- END of CODE