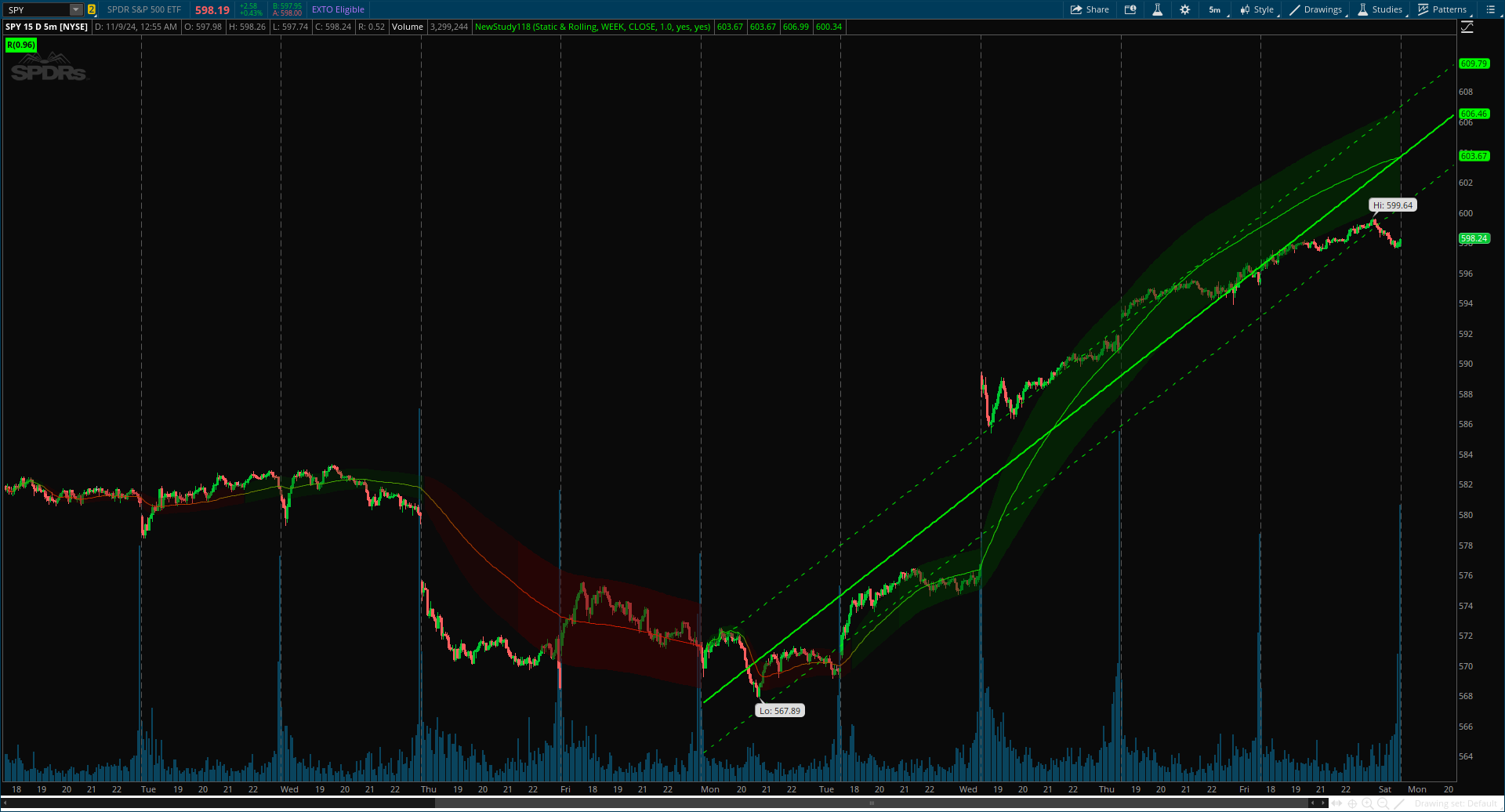
Author Message:
The Periodic Linear Regressions (PLR) indicator calculates linear regressions periodically (similar to the VWAP indicator) based on a user-set period (anchor).
This allows for estimating underlying trends in the price, as well as providing potential supports/resistances.
CODE:
Code:
#// Indicator for TOS
#// © LuxAlgo
#indicator('Periodic Linear Regressions [LuxAlgo]' , shorttitle='LuxAlgo - Periodic Linear Regressions'
# Converted by Sam4Cok@Samer800 - 11/2024
input showLabel = yes;
input Method = {"Static", default "Rolling", "Static & Rolling"}; # 'Method'
input AnchorPeriod = AggregationPeriod.WEEK;
input Source = close; # 'Source'
input Multiple = 1.0; # 'Multiple'
input ShowExtremities = yes; # , 'Show Extremities'
input extendStaticLinesToRight = no;
def na = Double.NaN;
def last = IsNaN(close);
def extend = extendStaticLinesToRight;
def current = GetAggregationPeriod();
def tf = Max(current, AnchorPeriod);
def bar = if !last then bar[1] + 1 else bar[1]; #if !last then BarNumber() else bar[1];
def n = if bar <=0 then 1 else bar;
def tgChage = if !last then close(Period = tf) else tgChage[1];
def ch = CompoundValue(1, tgChage!=tgChage[1], yes);
#-- Color
DefineGlobalColor("up", CreateColor(0, 52, 0));
DefineGlobalColor("dn", CreateColor(62, 0, 0));
script StDevTS {
input data = close;
input len = 12;
def length = Max(len , 1);
def avgData = (fold j = 0 to length with p do
p + GetValue(data, j)) / length;
def StDevTS = Sqrt((fold i = 0 to length with SD do
SD + Sqr(GetValue(data, i) - avgData)) / length);
plot out = StDevTS;
}
script covarianceTS {
input data1 = close;
input data2 = close;
input len = 12;
def length = Max(len , 1);
def avgData = (fold j = 0 to length with p do
p + GetValue(data1, j) * GetValue(data2, j)) / length;
def avgData1 = (fold j1 = 0 to length with p1 do
p1 + GetValue(data1, j1)) / length;
def avgData2 = (fold j2 = 0 to length with p2 do
p2 + GetValue(data2, j2)) / length;
plot CovarianceTS = avgData - avgData1 * avgData2;
}
#//add x & y values to array
def x1;
def Ex1;
def Ey1;
def Ex21;
def Exy1;
def aX1;
def aY1;
def Ex = Ex1[1] + n;
def Ey = Ey1[1] + Source;
def Ex2 = Ex21[1] + Power(n, 2);
def Exy = Exy1[1] + n * Source;
def x = Max(x1[1] + 1, 1);
def m = (x * Exy - Ex * Ey) / (x * Ex2 - power(Ex, 2));
def b = (Ey - m * Ex) / x;
def yMx = m * n + b;
def cov = covarianceTS(Source, n, x);
def x_var = Sqr(StDevTS(Source, x));
def a_ = cov / x_var;
def avgSrc = (fold j = 0 to x with q do
q + GetValue(Source, j)) / x;
def avgN = (fold i = 0 to x with p do
p + GetValue(n, i)) / x;
def b_ = avgSrc - a_ * avgN;
def r2 = power(cov / (StDevTS(Source, x) * StDevTS(n, x)), 2);
def rss = x_var - r2 * x_var;
def dist = sqrt(rss) * Multiple;
if ch {
Ex1 = 0;
Ey1 = 0;
Ex21 = 0;
Exy1 = 0;
x1 = 0;
aX1 = 0;
aY1 = 0;
} else {
Ex1 = Ex;
Ey1 = Ey;
Ex21 = Ex2;
Exy1 = Exy;
x1 = x;
aX1 = n;
aY1 = Source;
}
def R1 = cov / (StDevTS(Source, x) * StDevTS(n, x));
def R = if isNaN(R1) then 0 else R1;
def startBar = if ch then n else startBar[1];
def startSrc = if ch then Source else startSrc[1] ;
def lastDis = highestAll(inertiaAll(dist, 2, extendToRight = yes));
def cond = n > highestAll(inertiaAll(startBar, 2, extendToRight = yes));
def ln = if cond then Source else ln[1];
def lnU = if cond then Source + lastDis else lnU[1];
def lnD = if cond then Source - lastDis else lnD[1];
def endSrc = highestAll(inertiaAll(ln, 2, extendToRight = yes));
def plrRoll; def plrUpRoll; def plrDnRoll;
def plrStat; def plrUpStat; def plrDnStat;
Switch (Method) {
Case "Static & Rolling" :
plrRoll = if yMx then yMx else na;
plrUpRoll = if yMx and ShowExtremities then yMx + dist else na;
plrDnRoll = if yMx and ShowExtremities then yMx - dist else na;
plrStat = if cond then inertiaAll(ln, extendToRight = extend) else na;
plrUpStat = if cond and ShowExtremities then inertiaAll(lnU, extendToRight = extend) else na;
plrDnStat = if cond and ShowExtremities then inertiaAll(lnD, extendToRight = extend) else na;
Case "Static" :
plrRoll = na;
plrUpRoll = na;
plrDnRoll = na;
plrStat = if cond then inertiaAll(ln, extendToRight = extend) else na;
plrUpStat = if cond and ShowExtremities then inertiaAll(lnU, extendToRight = extend) else na;
plrDnStat = if cond and ShowExtremities then inertiaAll(lnD, extendToRight = extend) else na;
Default :
plrRoll = if yMx then yMx else na;
plrUpRoll = if yMx and ShowExtremities then yMx + dist else na;
plrDnRoll = if yMx and ShowExtremities then yMx - dist else na;
plrStat = na;
plrUpStat = na;
plrDnStat = na;
}
def endStat = if !isNaN(plrStat) then plrStat else endStat[1];
def staStat = if cond and !cond[1] then plrStat else staStat[1];
def colStatic1 = if (endStat > staStat) then 1 else 0;
def colStatic = if !isNaN(colStatic1) then colStatic1 else if isNaN(colStatic[1]) then 0 else colStatic[1];
def colRolling = if R > 1 then 4 else if R < -1 then 0 else (R + 1) * 2;
def upRolling = if plrUpRoll then plrUpRoll else na;
def dnRolling = if plrDnRoll then plrDnRoll else na;
plot midRolling = if plrRoll then plrRoll else na;
plot midStatic = if plrStat then plrStat else na;
plot upStatic = if plrUpStat then plrUpStat else na;
plot dnStatic = if plrDnStat then plrDnStat else na;
midRolling.AssignValueColor(CreateColor(255 - colRolling * 63, colRolling * 63, 0));
midStatic.SetLineWeight(2);
upStatic.SetStyle(Curve.SHORT_DASH);
dnStatic.SetStyle(Curve.SHORT_DASH);
midStatic.SetDefaultColor(Color.GRAY);
upStatic.SetDefaultColor(Color.GRAY);
dnStatic.SetDefaultColor(Color.GRAY);
midStatic.AssignValueColor(if colStatic>0 then Color.GREEN else Color.RED);
upStatic.AssignValueColor(if colStatic>0 then Color.GREEN else Color.RED);
dnStatic.AssignValueColor(if colStatic>0 then Color.GREEN else Color.RED);
AddCloud(if r >=0.65 then upRolling else na, dnRolling, GlobalColor("up"));
AddCloud(if r >=0 then upRolling else na, dnRolling, GlobalColor("up"));
AddCloud(if r <=-0.65 then upRolling else na, dnRolling, GlobalColor("dn"));
AddCloud(if r <=0 then upRolling else na, dnRolling, GlobalColor("dn"));
AddLabel(showLabel, "R (" + AsText(R) + ")", if R>0 then color.GREEN else Color.RED);
#-- END of CODE