Wave Trend Oscillator with Fractal Energy and divergence indicator
indicator is used to identify potential trend changes and entry/exit points by analyzing the cyclical movements of price and divergences.
Wave Trend is no lag but like all oscillators works well when a trade trends but is rife with false signals when it does not.
https://tos.mx/!WRYrx5cs
indicator is used to identify potential trend changes and entry/exit points by analyzing the cyclical movements of price and divergences.
Wave Trend is no lag but like all oscillators works well when a trade trends but is rife with false signals when it does not.
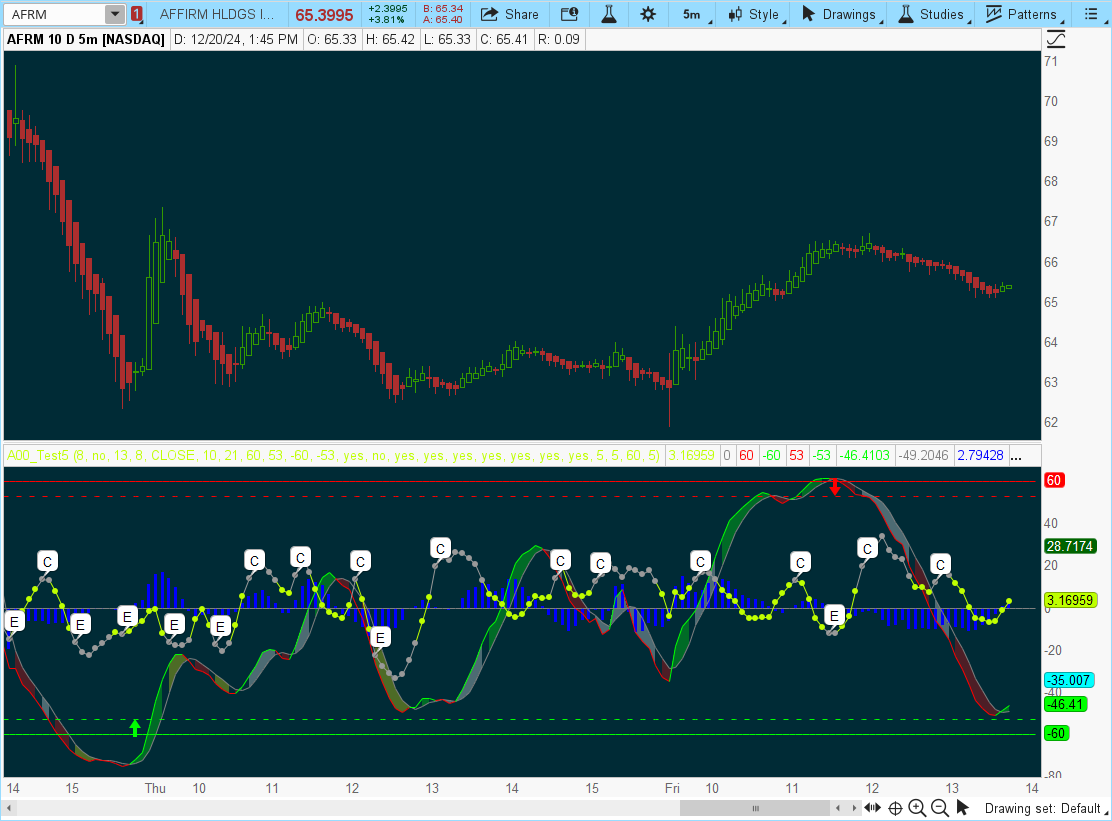
https://tos.mx/!WRYrx5cs
Code:
# RSI-Laguerre Self Adjusting With Fractal Energy Gaussian Price Filter
# Mobius
# V01.12.2016
# Both Fractal Energy and RSI are plotted. RSI in cyan and FE in yellow. Look for trend exhaustion in the FE and a reversal of RSI or Price compression in FE and an RSI reversal.
declare lower;
#Inputs:
input nFE = 8;#hint nFE: length for Fractal Energy calculation.
input AlertOn = no;
input Glength = 13;
input betaDev = 8;
input data = close;
def w = (2 * Double.Pi / Glength);
def beta = (1 - Cos(w)) / (Power(1.414, 2.0 / betaDev) - 1 );
def alpha = (-beta + Sqrt(beta * beta + 2 * beta));
def Go = Power(alpha, 4) * open +
4 * (1 – alpha) * Go[1] – 6 * Power( 1 - alpha, 2 ) * Go[2] +
4 * Power( 1 - alpha, 3 ) * Go[3] - Power( 1 - alpha, 4 ) * Go[4];
def Gh = Power(alpha, 4) * high +
4 * (1 – alpha) * Gh[1] – 6 * Power( 1 - alpha, 2 ) * Gh[2] +
4 * Power( 1 - alpha, 3 ) * Gh[3] - Power( 1 - alpha, 4 ) * Gh[4];
def Gl = Power(alpha, 4) * low +
4 * (1 – alpha) * Gl[1] – 6 * Power( 1 - alpha, 2 ) * Gl[2] +
4 * Power( 1 - alpha, 3 ) * Gl[3] - Power( 1 - alpha, 4 ) * Gl[4];
def Gc = Power(alpha, 4) * data +
4 * (1 – alpha) * Gc[1] – 6 * Power( 1 - alpha, 2 ) * Gc[2] +
4 * Power( 1 - alpha, 3 ) * Gc[3] - Power( 1 - alpha, 4 ) * Gc[4];
# Variables:
def o;
def h;
def l;
def c;
# Calculations
o = (Go + Gc[1]) / 2;
h = Max(Gh, Gc[1]);
l = Min(Gl, Gc[1]);
c = (o + h + l + Gc) / 4;
plot gamma = 100 *(Log(Sum((Max(Gh, Gc[1]) - Min(Gl, Gc[1])), nFE) /
(Highest(gh, nFE) - Lowest(Gl, nFE)))
/ Log(nFE))-50;
gamma.DefineColor("Con", CreateColor( 153, 153, 153));
gamma.DefineColor("EX", CreateColor( 153, 153, 153));
gamma.AssignValueColor(if gamma > 12 then gamma.Color("Con") else if gamma < -12 then gamma.Color("EX") else color.LIME);
def FE_Consolidation = gamma > 12 and gamma[1] < 12;
#AddLabel(FE_Consolidation, " Fractal Energy Consolidation ", Color.YELLOW);
def FE_Exhaustion = gamma < -12 and gamma[1] > -12;
#AddLabel(FE_Exhaustion, " Fractal Energy Exhaustion ", Color.YELLOW);
AddChartBubble(FE_Consolidation, gamma, "C", COLOR.WHITE);
AddChartBubble(FE_Exhaustion, gamma, "E", COLOR.WHITE);
gamma.SetPaintingStrategy(PaintingStrategy.LINE_VS_POINTS);
# End Code RSI_Laguerre Self Adjusting with Fractal Energy
#WT_LB Short Name TV
input Channel_Length = 10; #10
input Average_Length = 21; #10
input over_bought_1 = 60;
input over_bought_2 = 53;
input over_sold_1 = -60;
input over_sold_2 = -53;
input show_bubbles = yes;
input show_sec_bbls = no;
input show_alerts = yes;
def ap = hlc3;
def esa = ExpAverage(ap, Channel_Length);
def d = ExpAverage(AbsValue(ap - esa), Channel_Length);
def ci = (ap - esa) / (0.015 * d);
def tci = ExpAverage(ci, Average_Length);
def wt1 = tci;
def wt2 = SimpleMovingAvg(wt1, 4);
#def zero = 0;
plot zero = 0;
zero.SetDefaultColor( Color.GRAY );
plot obLevel1 = over_bought_1;
obLevel1.SetDefaultColor(Color.RED);
plot osLevel1 = over_sold_1;
osLevel1.SetDefaultColor(Color.GREEN);
plot obLevel2 = over_bought_2;
obLevel2.SetDefaultColor(Color.RED);
obLevel2.SetStyle(Curve.SHORT_DASH);
plot osLevel2 = over_sold_2;
osLevel2.SetDefaultColor(Color.GREEN);
osLevel2.SetStyle(Curve.SHORT_DASH);
plot wt1_1 = wt1;
wt1_1.assignvalueColor(if wt1 > wt2 then color.green else color.red);
plot wt2_1 = wt2;
wt2_1.SetDefaultColor(Color.gray);
#wt2_1.SetStyle(Curve.POINTS);
plot wt3 = (wt1 - wt2);
wt3.SetDefaultColor(Color.BLUE);
wt3.SetPaintingStrategy(PaintingStrategy.HISTOGRAM);
def signal1 = wt1 crosses above wt2 and wt1 < over_sold_2;
plot Signal = if signal1 then (signal1 * over_sold_2) else Double.NaN;
Signal.SetDefaultColor(Color.GREEN);
Signal.SetPaintingStrategy(PaintingStrategy.ARROW_UP);
Signal.SetLineWeight(3);
Signal.HideTitle();
def signal2 = wt1 crosses below wt2 and wt1 > over_bought_2;
plot Signal2_ = if signal2 then (signal2 * over_bought_2) else Double.NaN;
Signal2_.SetDefaultColor(Color.RED);
Signal2_.SetPaintingStrategy(PaintingStrategy.ARROW_DOWN);
Signal2_.SetLineWeight(3);
Signal2_.HideTitle();
def wt_1t = if gamma >= -12 and gamma <= 12 then wt1 else double.NaN;
def wt_1e = if gamma <= -12 then wt1 else double.NaN;
def wt_1c = if gamma >= 12 then wt1 else double.NaN;
def wt_2t = if gamma >= -12 and gamma <= 12 then wt2 else double.NaN;
def wt_2e = if gamma <= -12 then wt2 else double.NaN;
def wt_2c = if gamma >= 12 then wt2 else double.NaN;
addcloud(wt_1t,wt_2t, color.green, color.red);
addcloud(wt_1c,wt_2c, color.white, color.white);
addcloud(wt_1e,wt_2e, color.yellow, color.yellow);
#----Div-----------
input ShowLastDivLines = yes;
input ShowLastHiddenDivLines = yes;
input DivBull = yes; # "Plot Bullish"
input DivBear = yes; # "Plot Bearish"
input DivHiddenBull = yes; # "Plot Hidden Bullish"
input DivHiddenBear = yes; # "Plot Hidden Bearish"
input LookBackRight = 5; # "Pivot Lookback Right"
input LookBackLeft = 5; # "Pivot Lookback Left"
input MaxLookback = 60; # "Max of Lookback Range"
input MinLookback = 5; # "Min of Lookback Range"
def divSrc = wt1;
def na = Double.NaN;
script FindPivots {
input dat = close; # default data or study being evaluated
input HL = 0; # default high or low pivot designation, -1 low, +1 high
input lbL = 5; # default Pivot Lookback Left
input lbR = 1; # default Pivot Lookback Right
##############
def _nan; # used for non-number returns
def _BN; # the current barnumber
def _VStop; # confirms that the lookforward period continues the pivot trend
def _V; # the Value at the actual pivot point
##############
_BN = BarNumber();
_nan = Double.NaN;
_VStop = if !isNaN(dat) and lbr > 0 and lbl > 0 then
fold a = 1 to lbR + 1 with b=1 while b do
if HL > 0 then dat > GetValue(dat,-a) else dat < GetValue(dat,-a) else _nan;
if (HL > 0) {
_V = if _BN > lbL + 1 and dat == Highest(dat, lbL + 1) and _VStop
then dat else _nan;
} else {
_V = if _BN > lbL + 1 and dat == Lowest(dat, lbL + 1) and _VStop
then dat else _nan;
}
plot result = if !IsNaN(_V) and _VStop then _V else _nan;
}
#_inRange(cond) =>
script _inRange {
input cond = yes;
input rangeUpper = 60;
input rangeLower = 5;
def bars = if cond then 0 else bars[1] + 1;
def inrange = (rangeLower <= bars) and (bars <= rangeUpper);
plot retrun = inRange;
}
def pl_ = findpivots(divSrc,-1, LookBackLeft, LookBackRight);
def ph_ = findpivots(divSrc, 1, LookBackLeft, LookBackRight);
def pl = !isNaN(pl_);
def ph = !isNaN(ph_);
def pll = lowest(divSrc,LookBackLeft);
def phh = highest(divSrc,LookBackLeft);
def sll = lowest(low, LookBackLeft);
def shh = highest(high, LookBackLeft);
#-- Pvt Low
def plStart = if pl then yes else plStart[1];
def plFound = if (plStart and pl) then 1 else 0;
def vlFound1 = if plFound then divSrc else vlFound1[1];
def vlFound_ = if vlFound1!=vlFound1[1] then vlFound1[1] else vlFound_[1];
def vlFound = if !vlFound_ then pll else vlFound_;
def plPrice1 = if plFound then low else plPrice1[1];
def plPrice_ = if plPrice1!=plPrice1[1] then plPrice1[1] else plPrice_[1];
def plPrice = if !plPrice_ then sll else plPrice_;
#-- Pvt High
def phStart = if ph then yes else phStart[1];
def phFound = if (phStart and ph) then 1 else 0;
def vhFound1 = if phFound then divSrc else vhFound1[1];
def vhFound_ = if vhFound1!=vhFound1[1] then vhFound1[1] else vhFound_[1];
def vhFound = if !vhFound_ then phh else vhFound_;
def phPrice1 = if phFound then high else phPrice1[1];
def phPrice_ = if phPrice1!=phPrice1[1] then phPrice1[1] else phPrice_[1];
def phPrice = if !phPrice_ then sll else phPrice_;
#// Regular Bullish
def inRangePl = _inRange(plFound[1],MaxLookback,MinLookback);
def oscHL = divSrc > vlFound and inRangePl;
def priceLL = low < plPrice and divSrc <= 40;
def bullCond = plFound and oscHL and priceLL;
#// Hidden Bullish
def oscLL = divSrc < vlFound and inRangePl;
def priceHL = low > plPrice and divSrc <= 50;
def hiddenBullCond = plFound and oscLL and priceHL;
#// Regular Bearish
def inRangePh = _inRange(phFound[1],MaxLookback,MinLookback);
def oscLH = divSrc < vhFound and inRangePh;
def priceHH = high > phPrice and divSrc >= 60;;
def bearCond = phFound and oscLH and priceHH;
#// Hidden Bearish
def oscHH = divSrc > vhFound and inRangePh;
def priceLH = high < phPrice and divSrc >= 50;
def hiddenBearCond = phFound and oscHH and priceLH;
#------ Bubbles
def bullBub = DivBull and bullCond;
def HbullBub = DivHiddenBull and hiddenBullCond;
def bearBub = DivBear and bearCond;
def HbearBub = DivHiddenBear and hiddenBearCond;
addchartbubble(bullBub, divSrc, "R", color.GREEN, yes);
addchartbubble(bearBub, divSrc, "R", CreateColor(156,39,176), no);
addchartbubble(HbullBub, divSrc, "H", color.DARK_green, yes);
addchartbubble(HbearBub, divSrc, "H", color.DARK_red, no);
##### Lines
def bar = BarNumber();
#-- Bear Line
def lastPhBar = if ph then bar else lastPhBar[1];
def prePhBar = if lastPhBar!=lastPhBar[1] then lastPhBar[1] else prePhBar[1];
def priorPHBar = if bearCond then prePhBar else priorPHBar[1];
#-- Bull Line
def lastPlBar = if pl then bar else lastPlBar[1];
def prePlBar = if lastPlBar!=lastPlBar[1] then lastPlBar[1] else prePlBar[1];
def priorPLBar = if bullCond then prePlBar else priorPLBar[1];
def lastBullBar = if bullCond then bar else lastBullBar[1];
def lastBearBar = if bearCond then bar else lastBearBar[1];
def HighPivots = ph and bar >= HighestAll(priorPHBar) and bar <= HighestAll(lastBearBar);
def LowPivots = pl and bar >= HighestAll(priorPLBar) and bar <= HighestAll(lastBullBar);
def pivotHigh = if HighPivots then divSrc else na;
def pivotLow = if LowPivots then divSrc else na;
plot PlotHline = if ShowLastDivLines then pivotHigh else na;
PlotHline.EnableApproximation();
PlotHline.SetDefaultColor(Color.MAGENTA);
plot PlotLline = if ShowLastDivLines then pivotLow else na;
PlotLline.EnableApproximation();
PlotLline.SetDefaultColor(Color.CYAN);
#--- Hidden Lines
#-- Bear Line
def priorHPHBar = if hiddenBearCond then prePhBar else priorHPHBar[1];
#-- Bull Line
def priorHPLBar = if hiddenBullCond then prePlBar else priorHPLBar[1];
def lastHBullBar = if hiddenBullCond then bar else lastHBullBar[1];
def lastHBearBar = if hiddenBearCond then bar else lastHBearBar[1];
def HighHPivots = ph and bar >= HighestAll(priorHPHBar) and bar <= HighestAll(lastHBearBar);
def LowHPivots = pl and bar >= HighestAll(priorHPLBar) and bar <= HighestAll(lastHBullBar);
def pivotHidHigh = if HighHPivots then divSrc else na;
def pivotHidLow = if LowHPivots then divSrc else na;
plot PlotHBearline = if ShowLastHiddenDivLines then pivotHidHigh else na;
PlotHBearline.EnableApproximation();
PlotHBearline.SetDefaultColor(Color.DARK_RED);
plot PlotHBullline = if ShowLastHiddenDivLines then pivotHidLow else na;
PlotHBullline.EnableApproximation();
PlotHBullline.SetDefaultColor(Color.DARK_GREEN);
#-- END of CODE
Last edited by a moderator: