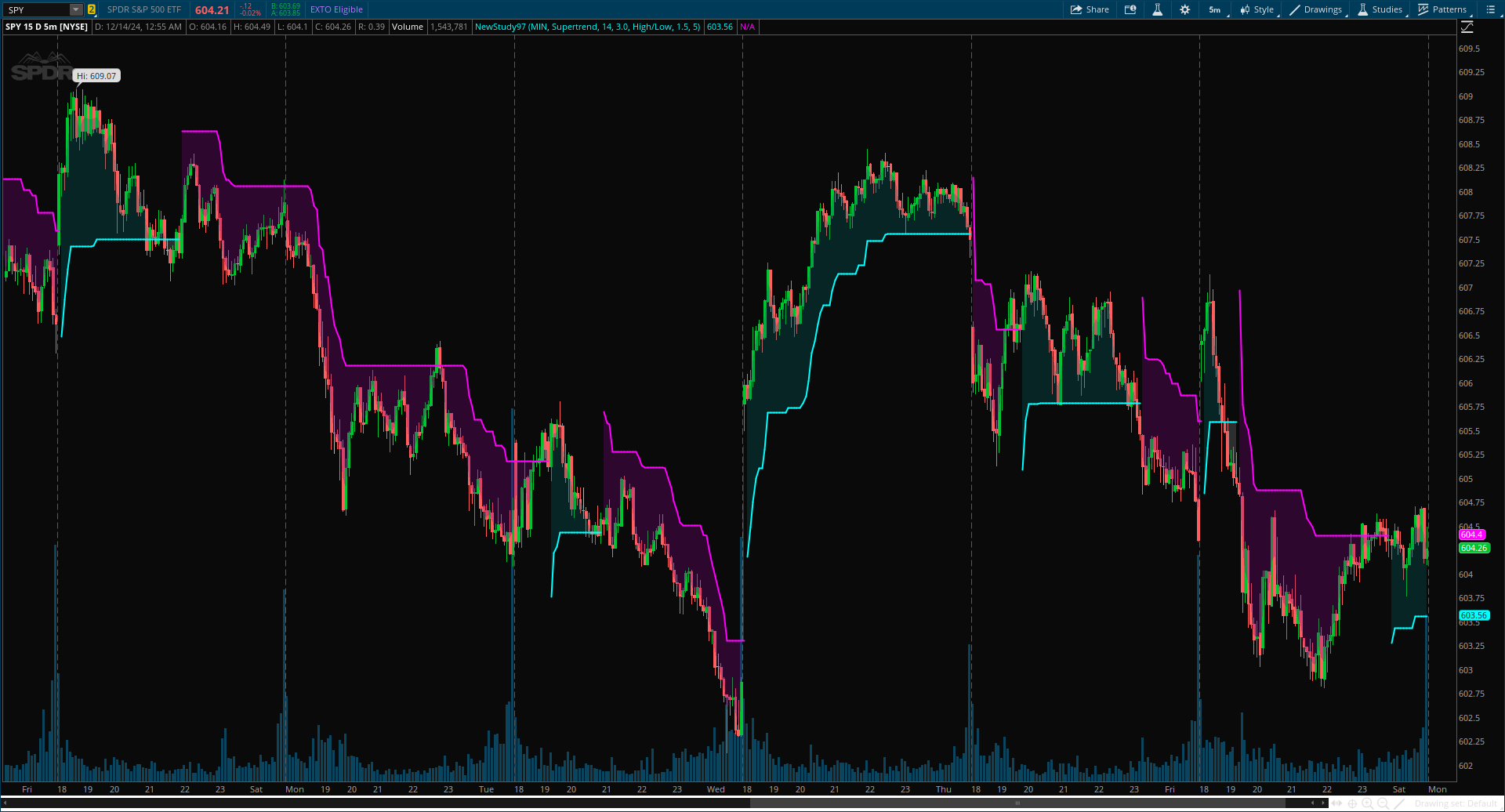
Author Message:
This script is an enhanced version of the classic Supertrend indicator. It incorporates an additional feature that ensures trend reversals are more reliable by introducing a Fakeout Index Limit and a Fakeout ATR Mult. This helps avoid false trend changes that could occur due to short-term price fluctuations or market noise.
CODE:
CSS:
#// Indicator for TOS
#// © EmreKb
#indicator("[EmreKb] Supertrend Fakeout", "ST Fakeout", overlay = true)
# Converted by Sam4Cok@Samer800 - 12/2024
input timeframe = AggregationPeriod.MIN;
input showOptions = {Default "Supertrend", "Bar Color", "Supertrend & Bar Color"};
input atrLength = 14; # "Supertrend ATR Length"
input atrMultiplier = 3.0; # "Supertrend ATR Mult"
input FakeoutBreakType = {default "High/Low", "Close"}; # "Fakeout Type"
input FakoutAtrMult = 1.5; # "Fakout ATR Mult"
input FakeoutCountLimit = 5; # "Fakeout Index Limit"
def na = Double.NaN;
def cap = GetAggregationPeriod();
def tf = Max(cap, timeframe);
def showST = showOptions==showOptions."Supertrend" or showOptions==showOptions."Supertrend & Bar Color";
def colorBars = showOptions==showOptions."Bar Color" or showOptions==showOptions."Supertrend & Bar Color";
def highSrc;
def lowSrc;
switch (FakeoutBreakType) {
case "Close" :
highSrc = close(Period = tf);
lowSrc = close(Period = tf);
default :
highSrc = high(Period = tf);
lowSrc = low(Period = tf);
}
Script f_supertrend {
input nATR = 1;
input atrMultiplier = 3.0;
input highSrc = high;
input lowSrc = low;
input FAKEOUT_INDEX_LIMIT = 5;
input FAKEOUT_ATR_MULT = 1.5;
def na = Double.NaN;
def src = (highSrc + lowSrc) / 2;
def lower = src - nATR * atrMultiplier;
def upper = src + nATR * atrMultiplier;
def state = {default ini, Bull, Bear};
def dnLine;
def upLine;
def fakeoutIndex;
def fakeoutSt;
def dn = if isNaN(dnLine[1]) then lower else if !dnLine[1] then lower else dnLine[1];
def up = if isNaN(upLine[1]) then upper else if !upLine[1] then upper else upLine[1];
def dnLine1 = if state[1] == state.Bull and dn > lower then dn else lower;
def upLine1 = if state[1] == state.Bear and up < upper then up else upper;
Switch (state[1]) {
Case Bull :
if lowSrc < dnLine[1] {
if (IsNaN(fakeoutIndex[1]) or IsNaN(fakeoutSt[1])) {
state = state[1];
upLine = upLine[1];
dnLine = dnLine[1];
fakeoutSt = upLine1;
fakeoutIndex = 0;
} else if (fakeoutIndex[1] >= FAKEOUT_INDEX_LIMIT) or ((dnLine[1] - lowSrc) > nATR * FAKEOUT_ATR_MULT) {
state = state.Bear;
upLine = fakeoutSt[1];
dnLine = dnLine1;
fakeoutSt = na;
fakeoutIndex = na;
} else {
state = state[1];
upLine = upLine[1];
dnLine = dnLine[1];
fakeoutSt = fakeoutSt[1];
fakeoutIndex = fakeoutIndex[1] + 1;
}
} else {
state = state[1];
upLine = upLine1;
dnLine = dnLine1;
fakeoutSt = na;
fakeoutIndex = na;
}
Case Bear:
if highSrc > upLine[1] {
if (IsNaN(fakeoutIndex[1]) or IsNaN(fakeoutSt[1])) {
state = state[1];
upLine = upLine[1];
dnLine = dnLine[1];
fakeoutSt = dnLine1;
fakeoutIndex = 0;
} else if (fakeoutIndex[1] >= FAKEOUT_INDEX_LIMIT) or ((highSrc - upLine[1]) > nATR * FAKEOUT_ATR_MULT) {
state = state.Bull;
upLine = upLine1;
dnLine = fakeoutSt[1];
fakeoutSt = na;
fakeoutIndex = na;
} else {
state = state[1];
upLine = upLine[1];
dnLine = dnLine[1];
fakeoutSt = fakeoutSt[1];
fakeoutIndex = fakeoutIndex[1] + 1;
}
} else {
state = state[1];
upLine = upLine1;
dnLine = dnLine1;
fakeoutSt = na;
fakeoutIndex = na;
}
Default :
dnLine = dnLine1;
upLine = upLine1;
state = state.Bull;
fakeoutSt = na;
fakeoutIndex = na;
}
plot st = if state == state.Bull then dnLine else upLine;
plot dir = if state == state.Bear then 0 else 1;
}
def tr = if isNaN(high(Period = tf)[1]) then (high(Period = tf) - low(Period = tf)) else
TrueRange(high(Period = tf), close(Period = tf), low(Period = tf));
def nATR = WildersAverage(tr, atrLength);
def trend = f_supertrend(nATR, atrMultiplier, highSrc, lowSrc, FakeoutCountLimit, FakoutAtrMult).dir;
def st = f_supertrend(nATR, atrMultiplier, highSrc, lowSrc, FakeoutCountLimit, FakoutAtrMult).st;
plot stBull = if showST and trend == 1 then st else na; # "Suptrend Bullish"
plot stBear = if !showST or trend == 1 then na else st; # "Suptrend Bearish"
stBull.SetLineWeight(2);
stBear.SetLineWeight(2);
stBull.SetDefaultColor(Color.CYAN);
stBear.SetDefaultColor(Color.MAGENTA);
AddCloud(if showST and trend == 1 then ohlc4 else na, st, CreateColor(0, 100, 100), Color.GRAY);
AddCloud(if !showST or trend == 1 then na else st , ohlc4, Color.PLUM, Color.GRAY);
#-- Bar Color
AssignPriceColor(if !colorBars then Color.CURRENT else
if trend == 1 then if close > st then Color.GREEN else Color.DARK_GREEN else
if close < st then Color.RED else Color.DARK_RED);
#-- END of CODE