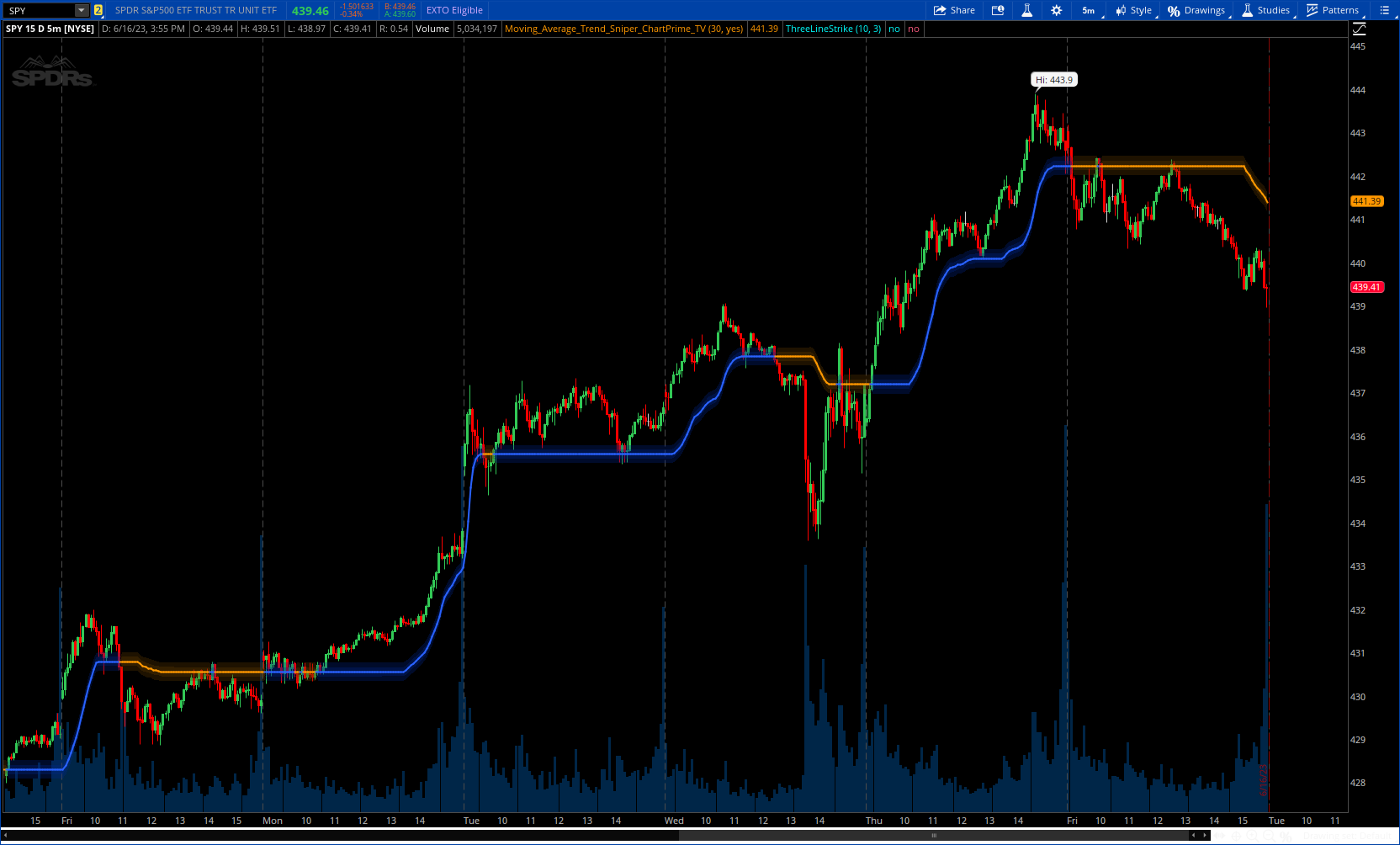
Author Message:
Moving Average Trend Sniper (MATS), a unique and powerful multi faceted tool. This moving average is designed to adapt to the ever-changing market conditions. MATS provides the ideal solution for traders looking to capitalize on market trends while accurately identifying support and resistance levels.
More Details : https://www.tradingview.com/v/iwEyBE2d/
CODE:
CSS:
#// This source code is subject to the terms of the Mozilla Public License 2.0 at https://mozilla.org/MPL/2.0/
#https://www.tradingview.com/v/iwEyBE2d/
#// © ChartPrime
#indicator("Moving Average Trend Sniper [ChartPrime]", "MATS [ChartPrime]", true)
# Converted by Sam4Cok@Samer800 - 06/2023
#// Custom cosh function
script cosh {
input x = close;
def cosh = (Exp(x) + Exp(-x)) / 2;
plot out = cosh;
}
#// Custom acosh function
script acosh {
input x = close;
def acosh = if x < 1 then Double.NaN else Log(x + Sqrt(x * x - 1));
plot out = acosh;
}
#// Custom sinh function
script sinh {
input x = close;
def sinh = (Exp(x) - Exp(-x)) / 2;
plot out = sinh;
}
#// Custom asinh function
script asinh {
input x = close;
def asinh = Log(x + Sqrt(x * x + 1));
plot out = asinh;
}
#// Custom inverse tangent function
script atan {
input x = close;
def pi = Double.Pi;
def atan = pi / 2 - ATan(1 / x);
plot out = atan;
}
#// Chebyshev Type I Moving Average
script chebyshevI {
input src = close;
input len = 30;
input ripple = 1;
def a = cosh(1 / len * acosh(1 / (1 - ripple)));
def b = sinh(1 / len * asinh(1 / ripple));
def g = (a - b) / (a + b);
def chebyshev = (1 - g) * src + g * chebyshev[1];
plot out = chebyshev;
}
script bool_to_float {
input source = close;
def bool = if source then 1 else 0;
plot out = bool;
}
script ema {
input source = close;
def count = count[1] + 1;
def ema = CompoundValue(1,(1.0 - 2.0 / (count + 1.0)) * ema[1] + 2.0 / (count + 1.0) * source, source);
plot out = ema;
}
script atan2 {
input y = 1;
input x = 1;
def pi = Double.Pi;
def angle;
if x > 0 {
angle = ATan(y / x);
} else
if x < 0 and y >= 0 {
angle = ATan(y / x) + pi;
} else
if x < 0 and y < 0 {
angle = ATan(y / x) - pi;
} else
if x == 0 and y > 0 {
angle = pi / 2;
} else
if x == 0 and y < 0 {
angle = -pi / 2;
} else {
angle = angle[1];
}
plot out = angle;
}
script degrees {
input source = close;
def pi = Double.Pi;
def deg = source * 180 / Pi;
plot out = deg;
}
script tra {
def tr = TrueRange(high, close, low);
def atr = ema(tr);
def slope = (close - close[10]) / (atr * 10);
def angle_rad = atan2(slope, 1);
def degrees = degrees(angle_rad);
def source = Average((if degrees > 0 then high else low), 2);
plot out = source;
}
script mats {
input source = close;
input length = 30;
# smooth = 0.
def hh = Highest(high, length);
def ll = Lowest(low, length);
def higher_high = Max(Sign(hh - hh[1]), 0);
def lower_low = Max(Sign((ll - ll[1]) * -1), 0);
def time_constant = Power(Average(bool_to_float(higher_high or lower_low), length), 2);
def smooth = CompoundValue(1, smooth[1] + time_constant * (source - smooth[1]), source);
def wilders_period = length * 4 - 1;
def atr = AbsValue(smooth[1] - smooth);
def ma_atr = ExpAverage(atr, wilders_period);
def delta_fast_atr = ExpAverage(ma_atr, wilders_period) * length * 0.4;
def result;# = 0.0
if smooth > result[1] {
if smooth - delta_fast_atr < result[1] {
result = result[1];
} else {
result = smooth - delta_fast_atr;
}
} else {
if smooth + delta_fast_atr > result[1] {
result = result[1];
} else {
result = smooth + delta_fast_atr;
}
}
plot out = result;
}
input length = 30; # "Length"
input enable_glow = yes; # "Enable Glow"
def na = Double.NaN;
#--- Color
#DefineGlobalColor("up", CreateColor(76, 175, 80));
#DefineGlobalColor("dn", CreateColor(255, 82, 82));
#DefineGlobalColor("dup", CreateColor(0, 80, 0));
#DefineGlobalColor("ddn", CreateColor(80, 0, 0));
DefineGlobalColor("up", CreateColor(41, 98, 255));
DefineGlobalColor("dn", CreateColor(255, 152, 0));
DefineGlobalColor("dup", CreateColor(0, 37, 139));
DefineGlobalColor("ddn", CreateColor(98, 58, 0));
def tr = TrueRange(high, close, low);
def mats = mats(tra(), length);
def col = if Average(close, 2) > mats then 1 else 0;
def atr_10 = ema(tr) / 2;
#alpha = color.new(color.black, 100)
def max = mats + atr_10;
def min = mats - atr_10;
def max1 = if enable_glow then mats + atr_10 / 2 else na;
def min1 = if enable_glow then mats - atr_10 / 2 else na;
plot center = mats;#, "Moving Average Trend Sniper", colour, editable = true)
def top = if enable_glow then max else na;#, "Moving Average Trend Sniper", alpha)
def bot = if enable_glow then min else na;#, "Moving Average Trend Sniper", alpha)
center.SetLineWeight(2);
center.AssignValueColor(if col then GlobalColor("up") else GlobalColor("dn"));
AddCloud(if col then max1 else mats, if col then mats else max1, GlobalColor("dup"), GlobalColor("ddn"));
AddCloud(if col then top else mats, if col then mats else top, GlobalColor("dup"), GlobalColor("ddn"));
AddCloud(if col then mats else min1, if col then min1 else mats, GlobalColor("dup"), GlobalColor("ddn"));
AddCloud(if col then mats else bot, if col then bot else mats, GlobalColor("dup"), GlobalColor("ddn"));
#--- END of CODE