Improved Extended-Hours Highlighter v2
by Steve Day
The following study is a replacement for the built-in Extended-Hours Highlighter.
It's primary purpose was to allow customization of the highlighting color, which in the built-in version is a fixed gray. I ended up expanding it to give separate colors to the Pre-Market & After-Hours sessions.
At the heart of it is a rather elegant (even if I do say so myself) time-zone offset calculation, which I created last year. By applying the time-zone to the UTC date/time, my study is able to automatically detect the (start of) Pre-Market & (end of) After-Hours sessions, with zero user input needed. (Their times do not have to be entered manually ... unlike even ToS's built-in scripts!).
As a secondary feature I added Market "Open" & "Close" vertical lines, as well as another vertical line which separates each day and optionally shows the date (like TradingView).
To use the custom-color highlighting you will need to disable the system's "Highlight Extended Hours" function, from each chart's "Settings -> Equities" tab.
ThinkOrSwim shared study: http://tos.mx/VwKPicC
Screenshots below, and code below that...
---------------------------------------- Screenshot #1/3 ----------------------------------------
Screenshot showing the study enabled.
---------------------------------------- Screenshot #2/3 ----------------------------------------
Screenshot of the study disabled, showing the system built-in Extended-Hours Highlighter (which has a non-adjustable gray color), and no separators or date for each day.
---------------------------------------- Screenshot #3/3 ----------------------------------------
Screenshot of the where to disable the built-in Extended-Hours Highlighter. This must be done for each chart that you want to use my highlighter with.
All 3 screenshots on one page: https://imgur.com/a/YPiLqnn
by Steve Day
The following study is a replacement for the built-in Extended-Hours Highlighter.
It's primary purpose was to allow customization of the highlighting color, which in the built-in version is a fixed gray. I ended up expanding it to give separate colors to the Pre-Market & After-Hours sessions.
At the heart of it is a rather elegant (even if I do say so myself) time-zone offset calculation, which I created last year. By applying the time-zone to the UTC date/time, my study is able to automatically detect the (start of) Pre-Market & (end of) After-Hours sessions, with zero user input needed. (Their times do not have to be entered manually ... unlike even ToS's built-in scripts!).
As a secondary feature I added Market "Open" & "Close" vertical lines, as well as another vertical line which separates each day and optionally shows the date (like TradingView).
To use the custom-color highlighting you will need to disable the system's "Highlight Extended Hours" function, from each chart's "Settings -> Equities" tab.
ThinkOrSwim shared study: http://tos.mx/VwKPicC
Screenshots below, and code below that...
---------------------------------------- Screenshot #1/3 ----------------------------------------
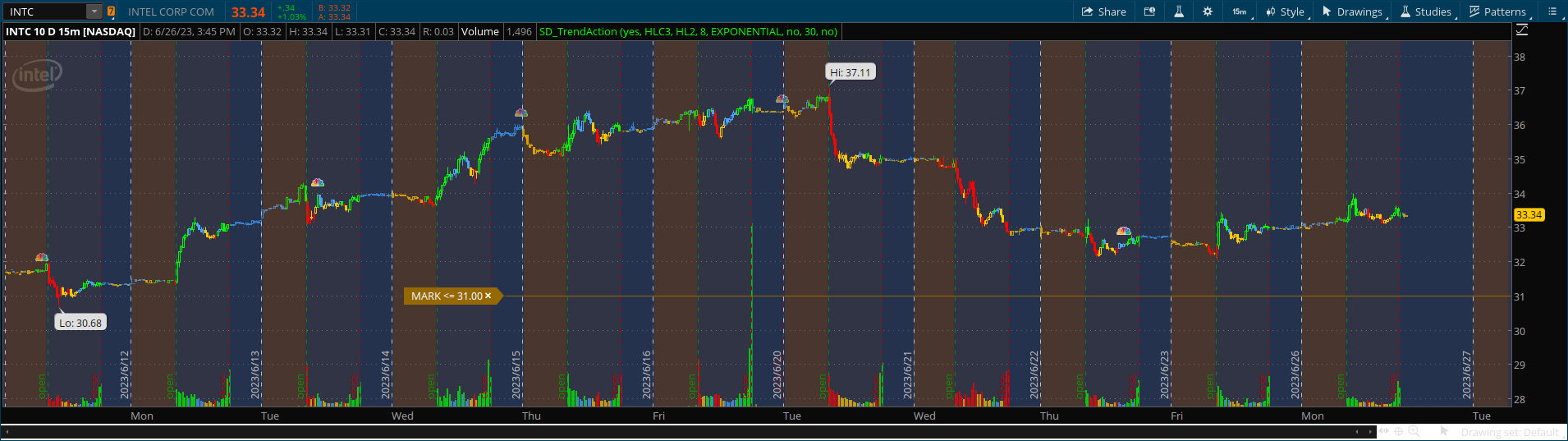
Screenshot showing the study enabled.
---------------------------------------- Screenshot #2/3 ----------------------------------------
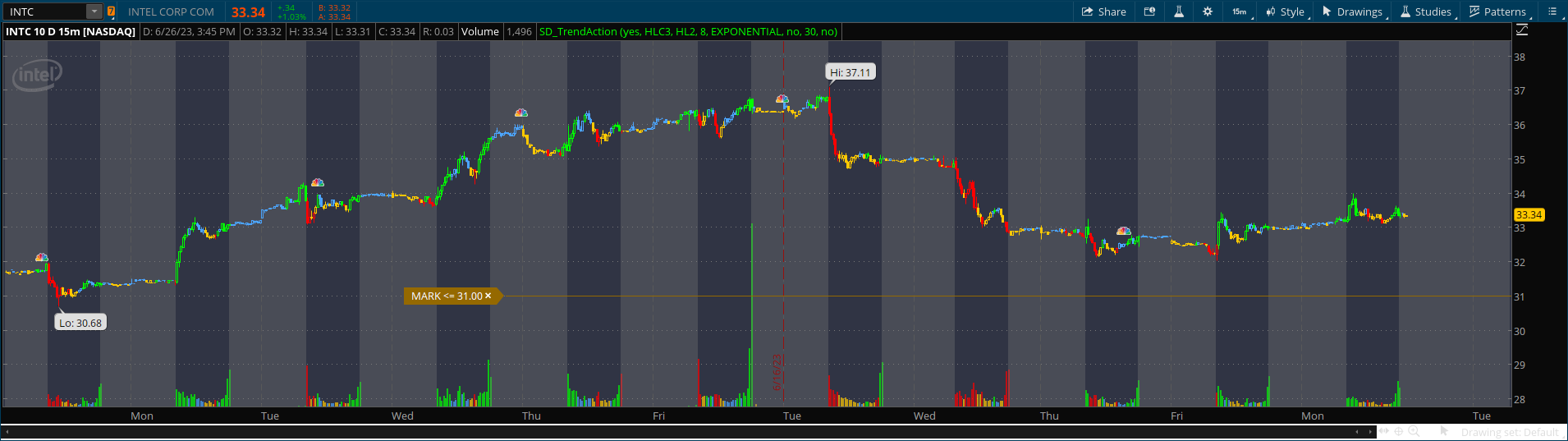
Screenshot of the study disabled, showing the system built-in Extended-Hours Highlighter (which has a non-adjustable gray color), and no separators or date for each day.
---------------------------------------- Screenshot #3/3 ----------------------------------------
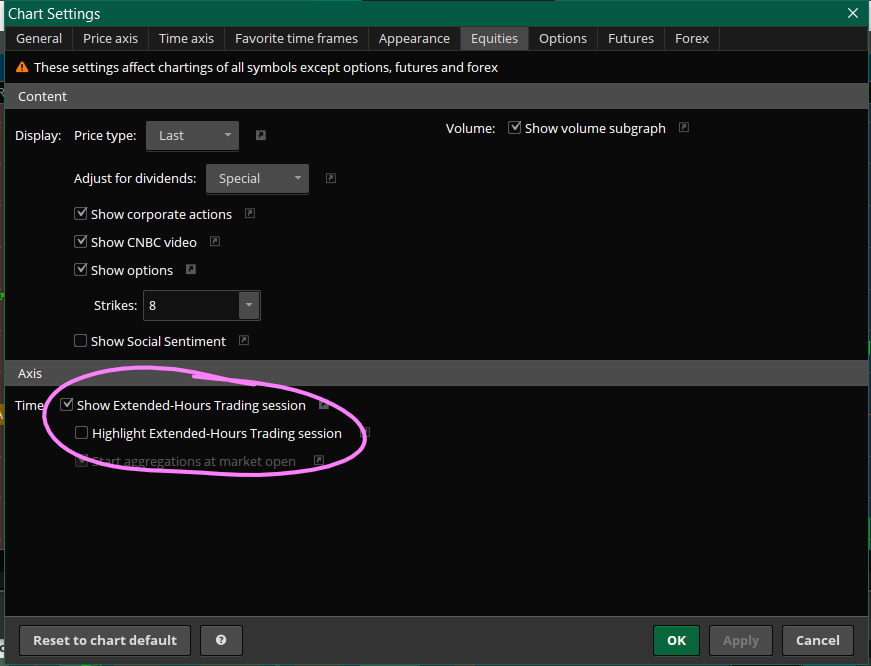
Screenshot of the where to disable the built-in Extended-Hours Highlighter. This must be done for each chart that you want to use my highlighter with.
All 3 screenshots on one page: https://imgur.com/a/YPiLqnn
Ruby:
#//# [SD] Improved Extended-Hours Highlighter v2.0.1
#==# -----------------------------------------------
#//# AUTHOR: Steve Day
#//# CREATED: 2023/06/15
#//# MODIFIED: 2023/06/26
#//# All Rights Reserved.
#..#
#!!# NOTICE: Feel free to share this script far and wide, as long as:
#!!# 1) You do not charge for, or "paywall" this script without my express permission.
#!!# 2) This full header is attached and I am credited as the author.
#..#
#..#
#//# PURPOSE: Built primarily to replace the built-in "Extended Hours Highlighter",
#//# which has a fixed highlight color (and fixed opacity).
#//# My version highlights the Pre-Market/After-Hours sessions separately.
#//# It will also display vertical lines to further highlight sessions.
#//# The colors are fully customizable, and the date is also shown on the day separator.
#//#
#//# SCREENS: https://imgur.com/a/YPiLqnn
#..#
#//# PROS: Fully customizable. Everything can be toggled on/off.
#//# Date displayed on day separators, like other charting platforms.
#//# Re-written from the ground up. Much more streamlined than v1.x
#//# CONS: It's forced to use "AddCloud" to highlight the extended sessions.
#//# AddCloud can "muddy" charts, due to how TOS blends colors.
#//# If this is a problem you can adjust the opacity of your colors,
#//# - or turn them off and rely on the vertical lines to mark sessions.
#//# The highlighting cloud starts and ends in the middle of a bar, there is no-
#//# - way around this, as it is a limitation of ThinkOrSwim.
#..#
#//# NOTE: I am open to freelance work. For offers, please contact: steve(at)steveday(dot)com
#..#
#//# Enjoy!
declare no_caching;
declare hide_on_daily;
declare once_per_bar;
#//# Inputs
input highlight_ExtSessions = yes;
input show_session_lines = yes;
input line_style = Curve.SHORT_DASH;
input show_market_text = yes;#hint show_market_text: 'show_market_text' toggles the visibility of the 'open' or 'close' text on the market open and market close lines.
input show_date = { "No" , "Normal", default "Zero Padded" };#hint show_date: Allows the user to select if they want the date displayed on each daily separation line. 'No' will display just the line without the date. 'Normal' will display the date, in a loose YYYY/mM/dD format, which leaves out any leading zeroes in the month or day numbers. The 'Zero Padded' option displays the date with leading zeroes added where necessary, so that it keeps the date a fixed length, in strict YYYY/MM/DD format.
#//# Colors
DefineGlobalColor("Ext.Session PM", CreateColor(128,64,0) );
DefineGlobalColor("Ext.Session AM", CreateColor(5,45,102) );
DefineGlobalColor("Day Separator" , Color.LIGHT_GRAY);
DefineGlobalColor("Market Open" , CreateColor(0,140,0) );
DefineGlobalColor("Market Close" , CreateColor(168,0,0) );
#//# Vars
def cap = GetAggregationPeriod();
def date = GetYYYYMMDD();
def time = GetTime();
def date_day = GetDayOfMonth(date);
def date_month = GetMonth();
def date_year = GetYear();
def barStart = time;
def barEnd = time + cap;
def msSinceMidnight = (time % AggregationPeriod.DAY);
def dayStart = time - msSinceMidnight;
def dayEnd = dayStart + AggregationPeriod.DAY;
def mktOpen = RegularTradingStart(date);
def mktClose = RegularTradingEnd(date);
#//# Session Logic
def isNewDay = date > date[1] and !IsNaN(date[1]);
def isMarketHours = time > mktOpen and time < mktClose;
def isAfterHours = time >= mktClose and time <= dayEnd;
def isPreMarket = time >= dayStart and time <= mktOpen;
#//# Session Highlighter Logic
def trig_ExtHours = if highlight_ExtSessions and (cap < AggregationPeriod.DAY) then
if isPreMarket then Double.POSITIVE_INFINITY
else if isAfterHours then Double.NEGATIVE_INFINITY
else Double.NaN
else Double.NaN;
#//# Highlight Sessions
AddCloud( trig_ExtHours , -trig_ExtHours
, GlobalColor("Ext.Session PM")
, GlobalColor("Ext.Session AM") );
#//# Vertical Line Logic
def draw_NewDay = show_session_lines and (cap < AggregationPeriod.DAY)
and isNewDay;
def draw_MktOpen = show_session_lines and (cap < AggregationPeriod.DAY)
and (!isMarketHours[1] and isMarketHours) and !isNewDay;
def draw_MktClose = show_session_lines and (cap < AggregationPeriod.DAY)
and (isMarketHours[1] and !isMarketHours) and !isNewDay;
#//# Draw Vertical Lines (Session Separators)
AddVerticalLine(draw_NewDay
, if show_date != show_date."No" then
AsPrice(date_year) + "/" +
if (show_date == show_date."Zero Padded") then
Concat( if date_month < 10 then "0" else "" , AsPrice(date_month)) + "/" +
Concat( if date_day < 10 then "0" else "" , AsPrice(date_day) )
else
AsPrice(date_month) + "/" +
AsPrice(date_day)
else ""
, GlobalColor("Day Separator") , line_style );
AddVerticalLine(draw_MktOpen
, if show_market_text then "open" else ""
, GlobalColor("Market Open") , line_style );
AddVerticalLine(draw_MktClose
, if show_market_text then "close" else ""
, GlobalColor("Market Close") , line_style );
Last edited: