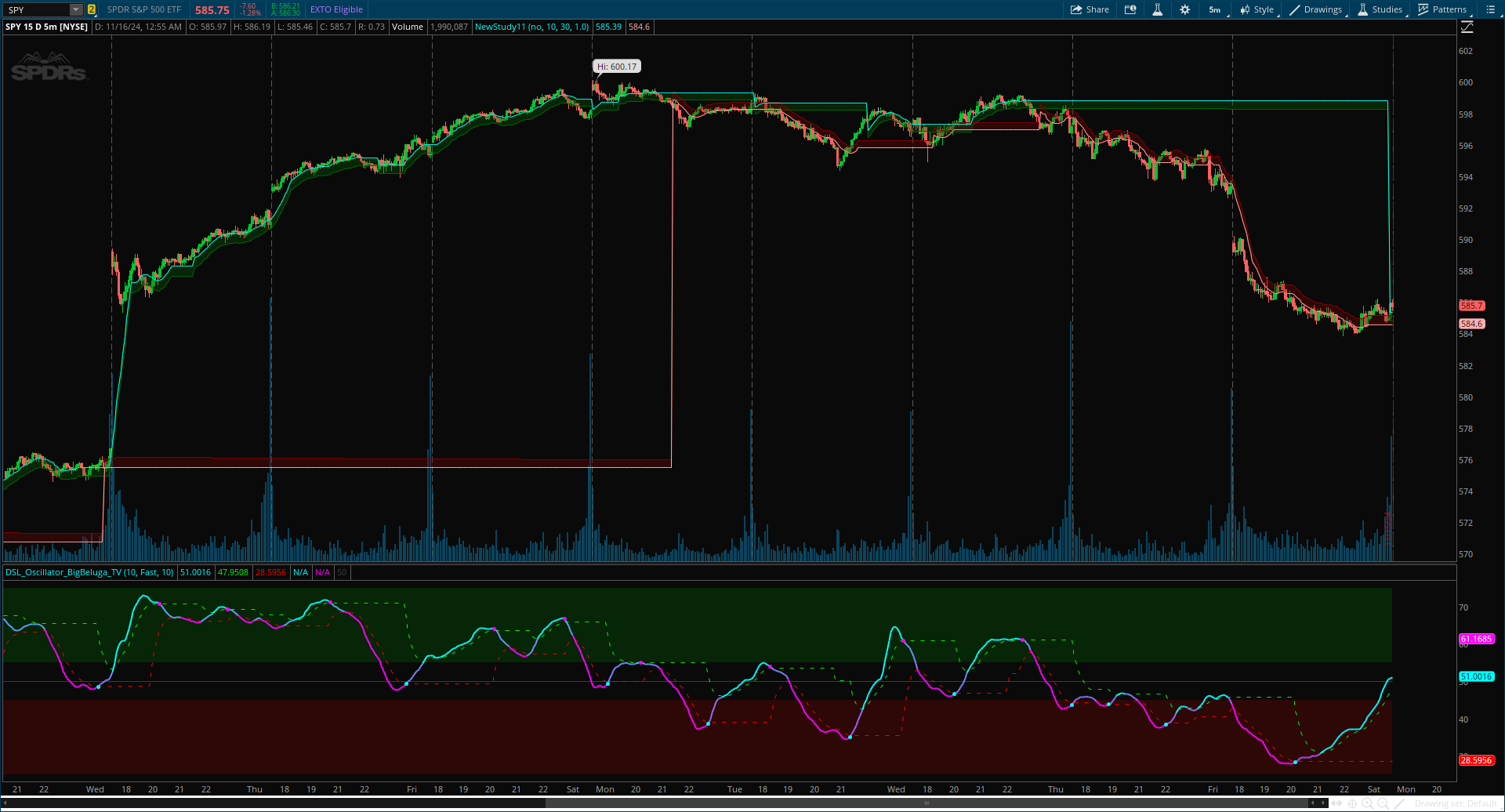
Author Message - Upper Study:
The DSL Trend Analysis [ChartPrime] indicator utilizes Discontinued Signal Lines (DSL) deployed directly on price, combined with dynamic bands, to analyze the trend strength and momentum of price movements. By tracking the high and low price values and comparing them to the DSL bands, it provides a visual representation of trend momentum, highlighting both strong and weakening phases of market direction.
Author Message - Lower Study:
The DSL (Discontinued Signal Lines) Oscillator is an advanced technical analysis tool that combines elements of the Relative Strength Index (RSI), Discontinued Signal Lines, and Zero-Lag Exponential Moving Average (ZLEMA). This versatile indicator is designed to help traders identify trend direction, momentum, and potential reversal points in the market.
CODE - Upper:
CSS:
#// Indicator for TOS
#// © ChartPrime
#indicator("DSL Trend Analysis [ChartPrime]", overlay = true)
# Converted by Sam4Cok@Samer800 - 11/2024
input colorBars = yes;
input dslLength = 10; # "Length" // Length for calculating DSL
input OffsetForThresholdLevels = 30; # "Offset" // Offset for threshold levels
input BandsWidth = 1.0; # "Bands Width" // Width for ATR-based bands
def na = Double.NaN;
def last = IsNaN(close);
#// 𝙄𝙉𝘿𝙄𝘾𝘼𝙏𝙊𝙍 𝘾𝘼𝙇𝘾𝙐𝙇𝘼𝙏𝙄𝙊𝙉𝙎
#// Function to calculate DSL lines based on price
script dsl_price {
input price = close;
input len = 10;
input offset = 30;
# // Initialize DSL lines as NaN (not plotted by default)
def sma = Average(price, len);
# // Dynamic upper and lower thresholds calculated with offset
def threshold_up = Highest(high, len)[offset];
def threshold_dn = Lowest(low, len)[offset];
# // Calculate the DSL upper and lower lines based on price compared to the thresholds
def dsl_up = if price > threshold_up then sma else dsl_up[1];
def dsl_dn = if price < threshold_dn then sma else dsl_dn[1];
# // Return both DSL lines
plot up = dsl_up;
plot dn = dsl_dn;
}
#// Function to calculate DSL bands based on ATR and width multiplier
script dsl_bands {
input dsl_up = high;
input dsl_dn = low;
input width = 1;
def atr = ATR(Length = 200) * width; #// ATR-based calculation for bands
def upper = dsl_up - atr; # // Upper DSL band
def lower = dsl_dn + atr; # // Lower DSL band
plot up = upper;
plot lo = lower;
}
#// Get DSL values based on the closing price
def dsl_up = dsl_price(close, dslLength, OffsetForThresholdLevels).up;
def dsl_dn = dsl_price(close, dslLength, OffsetForThresholdLevels).dn;
#// Calculate the bands around the DSL lines
def dsl_up1 = dsl_bands(dsl_up, dsl_dn, BandsWidth).up;
def dsl_dn1 = dsl_bands(dsl_up, dsl_dn, BandsWidth).lo;
#// Determine the trend color based on the relationship between price, DSL lines, and bands
def trend_col = if high > dsl_up1 and high < dsl_up and high > dsl_dn1 then 1 else
if low > dsl_dn and low < dsl_dn1 then -1 else
if high > dsl_up then 2 else if low < dsl_dn then -2 else 0;
#// 𝙑𝙄𝙎𝙐𝘼𝙇𝙄𝙕𝘼𝙏𝙄𝙊𝙉
# Bar Color
AssignPriceColor(if !colorBars then Color.CURRENT else
if trend_col == 2 then Color.GREEN else
if trend_col == 1 then Color.DARK_GREEN else
if trend_col ==-1 then Color.DARK_RED else
if trend_col ==-2 then Color.RED else Color.GRAY);
#// Plot the DSL lines on the chart
plot pu = if dsl_up then dsl_up else na; # "DSL Up"
plot pd = if dsl_dn then dsl_dn else na; # "DSL Down"
#// Plot the DSL bands
def pu1 = if dsl_up1 then dsl_up1 else na; # "DSL Upper Band"
def pd1 = if dsl_dn1 then dsl_dn1 else na; # "DSL Lower Band"
pu.SetDefaultColor(Color.CYAN);
pd.SetDefaultColor(Color.PINK);
AddCloud(pu, pu1, Color.DARK_GREEN, Color.DARK_GREEN, yes);
AddCloud(pd, pd1, Color.DARK_RED, Color.DARK_RED, yes);
#AddLabel(1, "Upper Band (" + AsDollars(dsl_up) + ")", Color.CYAN);
#AddLabel(1, "Lower Band (" + AsDollars(dsl_dn) + ")", Color.PINK);
# end of code
Code Lower :
CSS:
#// Indicator for TOS
#// © BigBeluga
#indicator("DSL Oscillator [BigBeluga]")
# Converted by Sam4Cok@Samer800 - 11/2024
Declare lower;
input dslLength = 10; #, "Length")
input dslLinesMode = {Default "Fast", "Slow"}; #])("Fast", "DSL Lines Mode", == "Fast" ? 2 : 1
input rsiLength = 10;
def na = Double.NaN;
def last = isNaN(close);
#// CALCULATIONS
#// Calculate RSI with a period of 10
def nRSI = rsi(Price = close,Length = rsiLength);
#// Zero-Lag Exponential Moving Average function
Script zlema {
input src = close;
input length = 10;
def lag = floor((length - 1) / 2);
def ema_data = 2 * src - src[lag];
def ema2 = ExpAverage(ema_data, length);
plot out = ema2;
}
#// Discontinued Signal Lines
Script dsl_lines {
input src = close;
input length = 10;
input dsl_mode = "Fast";
def dsl = if dsl_mode=="Fast" then 2 else 1;
def sma = Average(src, length);
def up; def dn;
def up1 = if up[1] then up[1] else 0;
def dn1 = if dn[1] then dn[1] else 0;
up = if (src > sma) then up1 + dsl / length * (src - up[1]) else up1;
dn = if (src < sma) then dn1 + dsl / length * (src - dn[1]) else dn1;
plot u = up;
plot d = dn;
}
#// Calculate DSL lines for RSI
def lvlu = dsl_lines(nRSI, dslLength, dslLinesMode).u;
def lvld = dsl_lines(nRSI, dslLength, dslLinesMode).d;
#// Calculate DSL oscillator using ZLEMA of the average of upper and lower DSL Lines
def dsl_osc = zlema((lvlu + lvld) / 2, 10);
#// Calculate DSL Lines for the oscillator
def level_up = dsl_lines(dsl_osc, 10, dslLinesMode).u;
def level_dn = dsl_lines(dsl_osc, 10, dslLinesMode).d;
#// PLOT
#// Plot the DSL oscillator
def col = if isNaN(dsl_osc) then 0 else
if dsl_osc > level_up then 100 else
if dsl_osc < level_dn then 0 else dsl_osc;
plot dslOsc = dsl_osc; #, color = color, linewidth = 2)
dslOsc.SetLineWeight(2);
dslOsc.AssignValueColor(CreateColor(255 - col*2.55, col * 2.55, 255));
#// Plot upper and lower DSL Lines
plot lvlUp = if level_up then level_up else na;
plot lvlDn = if level_dn then level_dn else na;
lvlUp.SetStyle(Curve.SHORT_DASH);
lvlDn.SetStyle(Curve.SHORT_DASH);
lvlUp.SetDefaultColor(Color.GREEN);
lvlDn.SetDefaultColor(Color.RED);
#// Detect crossovers for signal generation
def up = (dsl_osc Crosses Above level_dn) and dsl_osc < 55;
def dn = (dsl_osc Crosses Below level_up) and dsl_osc > 50;
#// Plot signals on the oscillator
plot upPoint = if up[-1] then dsl_osc else na;
plot dnPoint = if dn[-1] then dsl_osc else na;
upPoint.SetLineWeight(2);
dnPoint.SetLineWeight(2);
upPoint.SetStyle(Curve.POINTS);
dnPoint.SetStyle(Curve.POINTS);
upPoint.SetDefaultColor(Color.CYAN);
dnPoint.SetDefaultColor(Color.MAGENTA);
#// Plot horizontal lines for visual reference
def pos = Double.POSITIVE_INFINITY;
def neg = Double.NEGATIVE_INFINITY;
plot mid = if last then na else 50;
def ob = if last then na else 75;
def os = if last then na else 25;
mid.SetDefaultColor(Color.DARK_GRAY);
AddCloud(ob, mid+5, Color.DARK_GREEN);
AddCloud(mid-5, os, Color.DARK_RED);
#-- END of CODE